5 Tips for Mastering ANSI Terminal Colors | Unlock Python’s Color Secrets
ANSI Terminal Colors is a powerful technology that enables developers to set colors in the terminal, enhancing the visibility and organization of different types of output such as information, warnings, and errors. By using ANSI Terminal Colors controls, terminal output becomes more visually appealing, making the development process more intuitive and efficient.
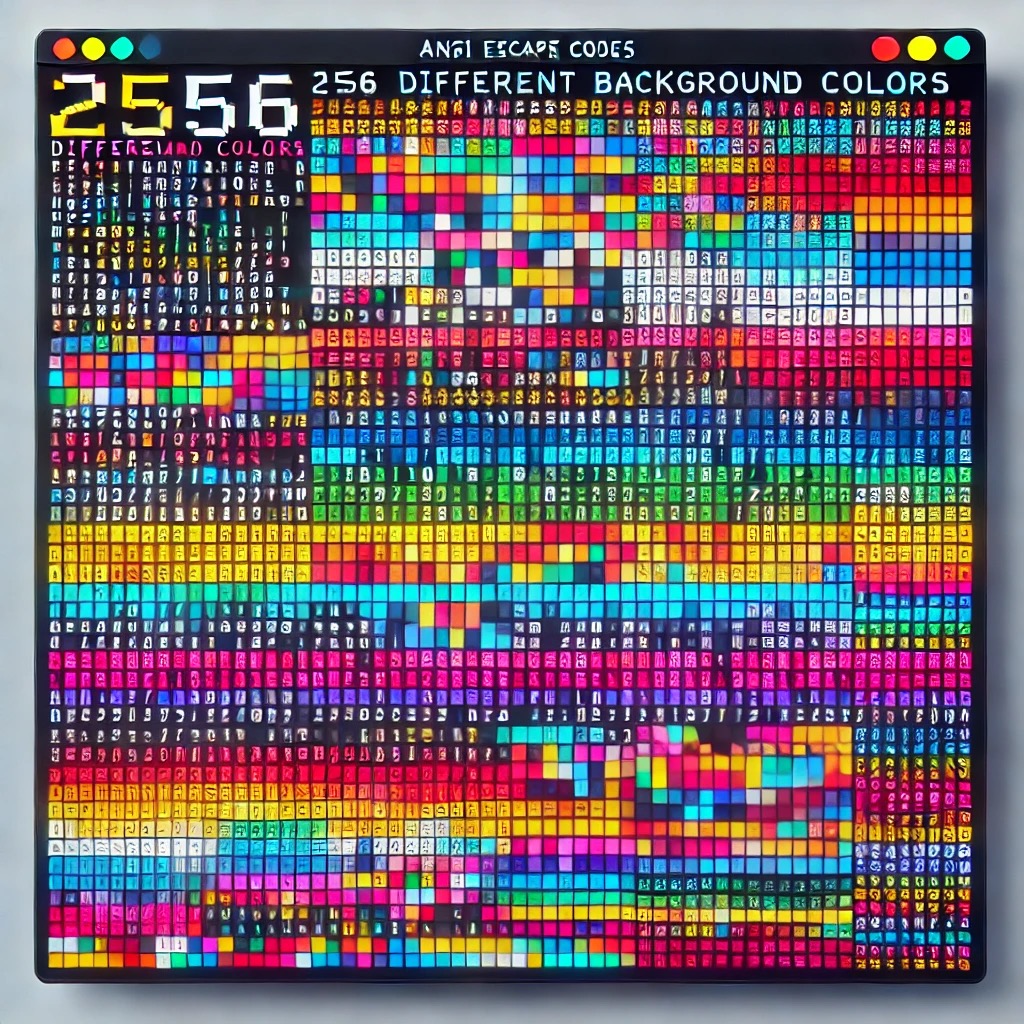
Contents
Introduction
ANSI (American National Standards Institute) is the organization responsible for establishing and promoting various national standards, one of which is terminal color control. ANSI escape sequences are a powerful tool for controlling display styles in the terminal, including colors, fonts, and cursor positioning.
Despite its utility, many developers are unfamiliar with the mechanics of ANSI Terminal Colors or how to leverage them to enhance terminal output. This article dives deep into the principles of ANSI terminal color control and demonstrates how Python can be used to unlock its potential, providing vibrant and dynamic terminal displays.
ANSI Terminal Colors Escape Sequences
ANSI escape sequences allow developers to control the display of terminal output with great precision.
Basic Structure
ESC[parameter1;parameter2;...m
- ESC: Escape character, represented as
\x1b
or\033
(octal). - [: Marks the start of the control sequence.
- Parameters: Define the style, foreground color, background color, or other display options.
- m: Marks the end of the sequence.
Common Parameter Combinations
Function | Foreground | Background | Style |
---|---|---|---|
Black | 30 | 40 | |
Red | 31 | 41 | |
Green | 32 | 42 | |
Yellow | 33 | 43 | |
Blue | 34 | 44 | |
Bold | 1 | ||
Underline | 4 |
Setting Up
- Install Python 3.x or higher.
- Ensure you have access to a terminal or command-line tool to run Python scripts.
Changing Text Color in Python
To output red text in Python using ANSI codes:
print("\033[31mThis is red text\033[0m")
\033[31m
: Sets the foreground color to red.\033[0m
: Resets the style to prevent affecting subsequent output.
Setting Foreground & Background Colors
To make color settings more flexible, you can write a function:
print("\033[33;44mYellow text on a blue background\033[0m")
Dynamic Color Configuration in Python
You can combine parameters to set both foreground and background colors:
def styled_text(text, fg=None, bg=None):
fg_code = f"3{fg}" if fg is not None else ""
bg_code = f"4{bg}" if bg is not None else ""
codes = ";".join(filter(None, [fg_code, bg_code]))
return f"\033[{codes}m{text}\033[0m"
print(styled_text("Dynamic colors", fg=2, bg=5)) # Green text on a magenta background
Adding Style Effects
Combine bold or underline effects with colors:
print("\033[1;31mBold red text\033[0m")
print("\033[4;34mUnderlined blue text\033[0m")
Color Codes and Examples
To display all 256 background colors on Unix-like (macOS or Linux) systems:
python3 -c "for i in range(256): print(f'\033[48;5;{i}m \033[0m', end='' if (i+1)%16 else '\n')"
For Windows, compatibility varies depending on the terminal used. Consider using PowerShell, Command Prompt, or third-party tools like Windows Terminal.
Complete Python Code
Here’s a full Python script demonstrating various ANSI terminal colors styles:
# Function to apply styled text with dynamic foreground and background colors
def styled_text(text, fg=None, bg=None):
fg_code = f"3{fg}" if fg is not None else ""
bg_code = f"4{bg}" if bg is not None else ""
codes = ";".join(filter(None, [fg_code, bg_code]))
return f"\033[{codes}m{text}\033[0m"
# Example 1: Basic Text Colors
print("\033[31mThis text is red\033[0m")
print("\033[32mThis text is green\033[0m")
print("\033[34mThis text is blue\033[0m")
# Example 2: Background Colors
print("\033[41mThis text has a red background\033[0m")
print("\033[42mThis text has a green background\033[0m")
print("\033[44mThis text has a blue background\033[0m")
# Example 3: Text Styles (Bold, Underline)
print("\033[1mThis text is bold\033[0m")
print("\033[4mThis text is underlined\033[0m")
# Example 4: Combining Colors and Styles
print("\033[1;31;47mBold red text on white background\033[0m")
print("\033[4;32;43mUnderlined green text on yellow background\033[0m")
# Example 5: 256-Color Mode
print("256-Color Background Examples:")
for i in range(16, 32):
print(f"\033[48;5;{i}m {i:3} \033[0m", end=" ")
print() # Move to the next line
# Example 6: RGB True Color (24-bit)
print("\033[38;2;255;105;180mPink text\033[0m")
print("\033[48;2;70;130;180mText with Steel Blue background\033[0m")
# Example 7: Using the styled_text function with dynamic colors
print(styled_text("Dynamic colors", fg=2, bg=5)) # Green text on a magenta background
For cross-platform compatibility, consider using libraries like colorama
to ensure consistent behavior on Windows OS.
Conclusion
ANSI Terminal Colors are a valuable tool for enhancing the visual and interactive experience of terminal applications. By understanding ANSI escape sequences, you can customize terminal output effectively, improving readability and user engagement. Master these techniques to build more dynamic and visually appealing terminal applications, enhancing your development workflow.