Revealing the Hidden Skills of ESP32 UART Events | Smarter Serial Communication Made Easy
ESP32 UART Events are a ubiquitous communication tool in embedded development, allowing your ESP32 to seamlessly interact with external modules. But have you truly unlocked the full potential of ESP32 UART Events? In the ESP-IDF framework, the UART event-driven mechanism hides numerous powerful and often ‘hidden’ features. Today, we will explore these secrets and elevate the intelligence of your communication system.
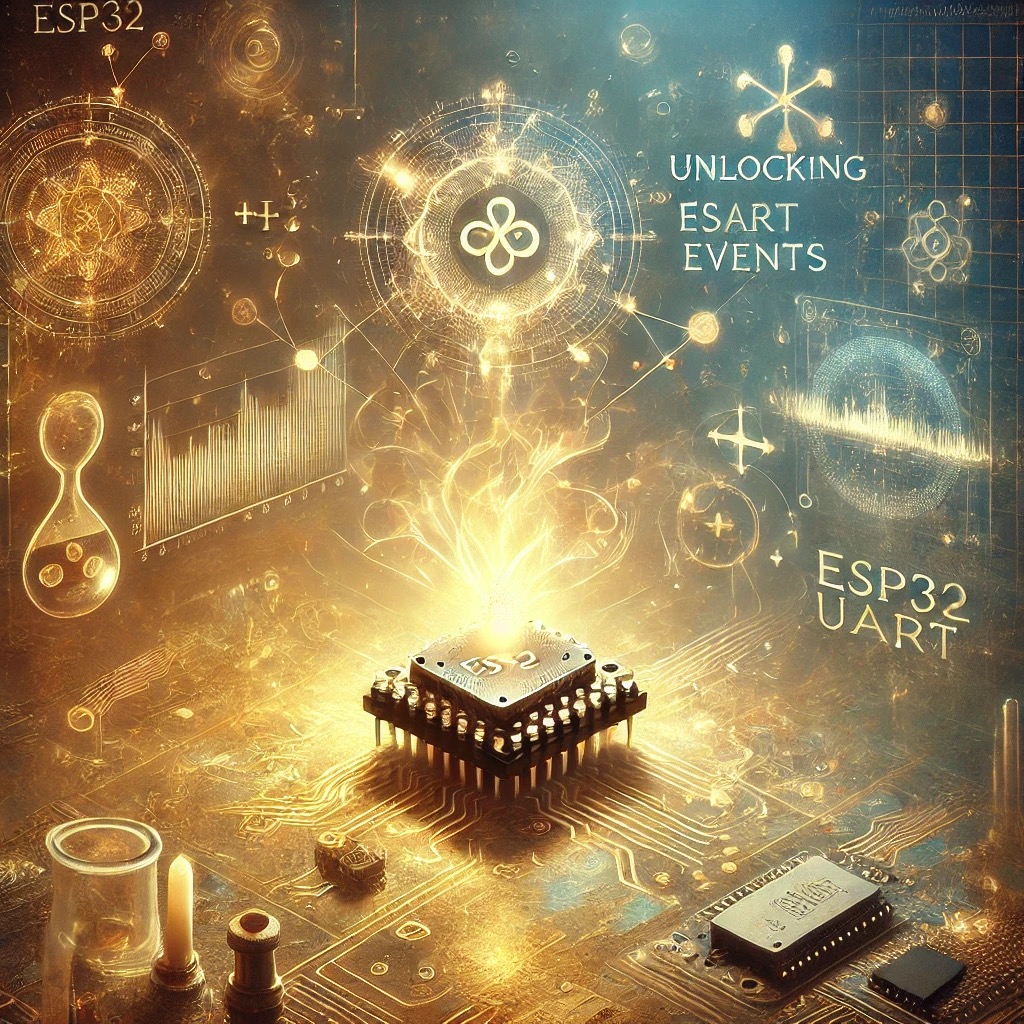
Contents
Introduction
n embedded development, ESP32 UART Events provide a powerful event-driven mechanism to efficiently handle various scenarios in serial communication. As a high-performance SoC, the ESP32’s built-in UART features can not only perform basic data transmission but also detect multiple events such as data reception, buffer overflow, and pattern matching. These events, when handled properly, can significantly enhance communication stability and efficiency.
Through the event-driven model, developers can not only respond promptly to errors and anomalies but also implement smarter operations based on the flow of data. In this guide, we’ll delve into how to utilize UART events on ESP32, uncover its hidden features, and elevate the intelligence of your communication system.
What Is the ESP32 UART Events ?
The UART driver in ESP32 is equipped with a range of event types that can react to real-time conditions such as data reception, buffer status changes, or communication errors. These events are defined by the uart_event_type_t
enum and include:
- UART_DATA: Data received.
- UART_BUFFER_FULL: RX buffer full.
- UART_FIFO_OVF: FIFO overflow.
- UART_BREAK: Break signal detected.
- UART_PATTERN_DET: Specific pattern detected.
- UART_FRAME_ERR and UART_PARITY_ERR: Communication errors.
The power of these events lies in their ability to make the UART driver “aware,” enabling quick reactions based on the current communication state, thereby improving reliability and efficiency.
Why Use ESP32 UART Events ?
Traditional UART communication is blocking and requires the developer to manually handle every step of data processing. The event-driven model offers several advantages:
- Non-blocking operations: Prevents the CPU from idly waiting for data.
- Real-time error handling: Quickly responds to overflow, errors, and other anomalies.
- Efficient pattern detection: Simplifies handling of custom communication protocols.
In embedded development, this means your system can “sense” and “adapt” to dynamically changing communication needs, resulting in improved stability.
Development Environment
Before starting your programming, make sure to complete the following preparations:
- Install ESP-IDF (version 4.4 or higher): ESP-IDF is the official development framework for programming the ESP32, and it supports multiple operating systems such as Windows, macOS, and Linux.
- ESP32 Development Board: An ESP32 board is required.
- Other reference articles.
Initializing UART Configuration
Below is a complete example of ESP32 UART events handling, covering UART initialization, setting up an event queue, and creating an event-handling task.
Step 1: UART Initialization
#include <stdio.h>
#include <string.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/uart.h"
#include "esp_log.h"
static const char *TAG = "uart_events"; // Define a tag for logging
// Define UART port and buffer sizes
#define EX_UART_NUM UART_NUM_0
#define BUF_SIZE (1024)
#define PATTERN_CHR_NUM (3) // Define the number of consecutive characters to be detected for a pattern
static QueueHandle_t uart0_queue; // Queue to handle UART events
// UART initialization function
void uart_init()
{
// Configure UART parameters
uart_config_t uart_config = {
.baud_rate = 115200, // Set baud rate
.data_bits = UART_DATA_8_BITS, // Set data bits to 8
.parity = UART_PARITY_DISABLE, // Disable parity check
.stop_bits = UART_STOP_BITS_1, // Set stop bits to 1
.flow_ctrl = UART_HW_FLOWCTRL_DISABLE, // Disable hardware flow control
.source_clk = UART_SCLK_APB, // Use APB clock
};
// Install UART driver and configure UART parameters
uart_driver_install(EX_UART_NUM, BUF_SIZE * 2, BUF_SIZE * 2, 20, &uart0_queue, 0);
uart_param_config(EX_UART_NUM, &uart_config); // Apply UART configuration
// Set UART pins (use default UART0 pins)
uart_set_pin(EX_UART_NUM, UART_PIN_NO_CHANGE, UART_PIN_NO_CHANGE, UART_PIN_NO_CHANGE, UART_PIN_NO_CHANGE);
// Enable UART pattern detection: detect 3 consecutive '+' characters as a pattern
uart_enable_pattern_det_baud_intr(EX_UART_NUM, '+', PATTERN_CHR_NUM, 9, 0, 0);
// Reset the pattern queue to record up to 20 pattern positions
uart_pattern_queue_reset(EX_UART_NUM, 20);
}
Event Handling Task
Step 2: Create a Task to Handle ESP32 UART Events
// UART event handling task
static void uart_event_task(void *pvParameters)
{
uart_event_t event; // Variable to hold UART event
uint8_t* dtmp = (uint8_t*) malloc(BUF_SIZE); // Allocate memory for receiving data
for(;;) {
// Wait for UART events
if(xQueueReceive(uart0_queue, (void * )&event, portMAX_DELAY)) {
bzero(dtmp, BUF_SIZE); // Clear the buffer
ESP_LOGI(TAG, "uart[%d] event:", EX_UART_NUM); // Log the event
switch(event.type) {
case UART_DATA:
// Handle UART data received event
ESP_LOGI(TAG, "[UART DATA]: %d bytes received", event.size);
uart_read_bytes(EX_UART_NUM, dtmp, event.size, portMAX_DELAY); // Read received data
uart_write_bytes(EX_UART_NUM, (const char*) dtmp, event.size); // Echo the received data
ESP_LOGI(TAG, "[DATA EVT]: Data echoed back: %s", dtmp); // Log the echoed data
break;
case UART_FIFO_OVF:
// Handle UART FIFO overflow event
ESP_LOGI(TAG, "FIFO overflow detected");
uart_flush_input(EX_UART_NUM); // Clear the input buffer
break;
case UART_BUFFER_FULL:
// Handle UART buffer full event
ESP_LOGI(TAG, "Ring buffer is full");
uart_flush_input(EX_UART_NUM); // Clear the input buffer
break;
case UART_PATTERN_DET:
// Handle UART pattern detection event
ESP_LOGI(TAG, "Pattern detected");
uart_get_buffered_data_len(EX_UART_NUM, &event.size); // Get the buffered data length
ESP_LOGI(TAG, "Buffered data size: %d", event.size); // Log the size of buffered data
break;
default:
// Handle other unknown UART events
ESP_LOGI(TAG, "Unknown uart event type: %d", event.type);
break;
}
}
}
free(dtmp); // Free the allocated memory
dtmp = NULL; // Set pointer to NULL
vTaskDelete(NULL); // Delete the task once done
}
Main Program
Step 3: Integrate Initialization and Task in Main
void app_main(void)
{
// Initialize UART
uart_init();
// Create UART event handling task
xTaskCreate(uart_event_task, "uart_event_task", 2048, NULL, 12, NULL);
}
Code Explanation
UART Initialization (uart_init
):
- Configure UART parameters like baud rate, data bits, stop bits, etc.
- Enable pattern detection to trigger
UART_PATTERN_DET
when three consecutive+
characters are detected. - Configure the pattern queue: Records up to 20 detected pattern positions.
Event Handling Task (uart_event_task
):
- uart_driver_install: Used to install the UART driver and create an event queue to receive events from UART.
- xQueueReceive: Blocks and waits to receive events. It receives and processes all events coming from UART.
- uart_read_bytes: Used to read the received data and process it.
- UART_DATA: Reads data and echoes it back while printing the received data.
- UART_FIFO_OVF and UART_BUFFER_FULL: Handles buffer overflow situations to prevent data loss.
- UART_PATTERN_DET: The event can be used to detect specific byte patterns.
Logging (esp_log
):
- Provides detailed logging to monitor UART states for debugging and development.
Why use UART pattern detection:
The pattern detection feature (UART_PATTERN_DET) can significantly simplify the implementation of specific character matching, especially in the following scenarios:
- Protocol parsing: Detecting start or end markers in a message.
- Data segmentation: Splitting the data stream into multiple segments based on a specific pattern.
- Diagnostics and debugging: Real-time monitoring of specific character sequences in the data flow.
Conclusion
The ESP32 UART Events mechanism offers an efficient and flexible way to handle serial communication. By categorizing and responding to events, you can manage data reception, overflow, and pattern detection with ease. This mechanism not only reduces the CPU workload but also enhances communication reliability and scalability. We hope this guide helps you better understand ESP32 UART Events and empowers your projects with smarter communication capabilities.