Master Arduino ESP32 Callback Functions | Handling Button Events Made Easy!
ESP32 Callback functions are a powerful feature in embedded development, enabling developers to simplify code and improve efficiency when handling event-driven applications. Especially for hardware interactions like button events, callback functions make your code more readable and scalable. This article introduces how to use callback functions in the Arduino IDE to handle button events efficiently. It also explores the concept of callback functions, their pros and cons, and how to apply them in real-world development.
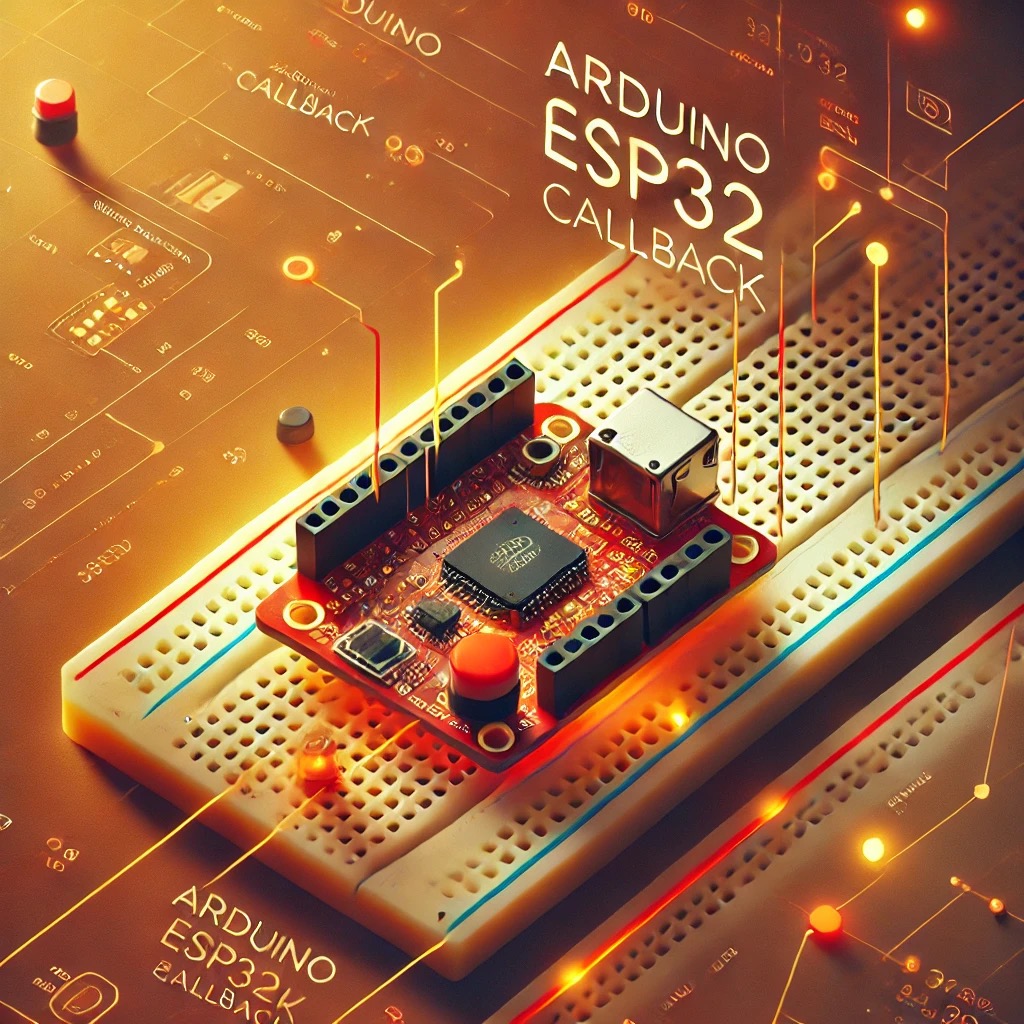
Contents
Introduction
When developing ESP32 projects, efficiently handling hardware events—especially button events—can be challenging. Traditional methods often involve complex polling techniques, which increase code complexity and reduce system performance. However, by utilizing ESP32 Callback functions, you can simplify hardware event handling significantly.
ESP32 Callback functions are a common method in event-driven programming, allowing developers to delegate control to a predefined function when a specific event occurs. This not only reduces code repetition but also improves program readability and maintainability.
What is a Callback Function?
In programming, a callback function is a function passed as an argument to another function, allowing it to be executed when a particular event or condition occurs. Essentially, the system “calls back” the specified function to handle the event.
For ESP32 development, callback functions are commonly used for asynchronous events such as button presses, signal reception, or other hardware interactions. The GPIO (General Purpose Input/Output) pins on the ESP32 can be configured to trigger interrupt events, which activate callback functions upon button presses or releases.
In Arduino ESP32 development, setting up callback functions is straightforward. By doing so, you can separate button event handling code from other parts of the program logic, making your code more modular and maintainable.
Pros and Cons of Callback Functions
Pros:
- Simplifies Code: Callback functions automatically trigger the appropriate logic when an event occurs, eliminating the need for extensive polling or conditional checks.
- Asynchronous Processing: Callback functions are executed when the event occurs, avoiding unnecessary delays and enhancing program responsiveness.
- Improves Readability and Maintainability: Abstracting event-handling logic into separate functions makes the code more concise and easier to understand, especially when dealing with multiple asynchronous events.
- Flexibility: Developers can assign different handling logic to various events, significantly increasing the program’s flexibility and scalability.
Cons:
- Error Handling: Asynchronous execution can make error handling less intuitive compared to synchronous programming, complicating debugging.
- Debugging Challenges: Debugging callback functions can be difficult due to their asynchronous nature, especially in complex scenarios.
- Overuse May Increase Complexity: Excessive use of callback functions can lead to fragmented code, making it harder to understand and maintain.
Development Environment
Before starting your programming, make sure to complete the following preparations:
- Arduino IDE: Install the Arduino IDE and set up the ESP32 development environment. The IDE supports multiple operating systems, including Windows, macOS, and Linux.
- ESP32 Development Board: An ESP32 board is required.
Arduino ESP32 Callback for Button Events
Using ESP32 callback functions to handle button events is straightforward. Set up the GPIO pins and register an interrupt callback function. When the button is pressed or released, the corresponding handling function is automatically triggered.
const int buttonPin = 15; // GPIO pin connected to the button
volatile bool buttonPressed = false; // Variable to store the button state
// Callback function for button press events
void IRAM_ATTR handleButtonPress() {
buttonPressed = true; // Set the button pressed state
}
void setup() {
Serial.begin(115200); // Start serial communication
pinMode(buttonPin, INPUT_PULLUP); // Configure the button pin as input with an internal pull-up resistor
// Attach the interrupt and register the callback function
attachInterrupt(buttonPin, handleButtonPress, FALLING);
Serial.println("Press the button to trigger an event...");
}
void loop() {
if (buttonPressed) {
Serial.println("Button pressed!");
buttonPressed = false; // Reset the button state for the next detection
}
delay(100); // Debounce delay
}
Code Explanation
- GPIO Configuration: The
pinMode
function configures the GPIO pin as an input and enables the internal pull-up resistor. This ensures a low signal (FALLING) when the button is pressed. - Interrupt Registration: The
attachInterrupt
function binds the button press event to the ESP32 Callback functionhandleButtonPress
. When GPIO pin 15 detects a falling edge (button press), the callback function is triggered. - Callback Function: The
handleButtonPress
function is the core of the ESP32 Callback mechanism, setting thebuttonPressed
flag totrue
and allowing the main loop to respond to the button press event.
Output
When you run this program, the Serial Monitor will display the following output, assuming you press and release the button:
- Startup Output:
When the program initializes, the following message is displayed:Press the button to trigger an event...
- Button Press Output:
Each time the button is pressed, thehandleButtonPress
callback setsbuttonPressed
totrue
, triggering the condition in the main loop. For a single button press, the Serial Monitor will display:Button pressed!
- Continuous Input Behavior:
If you press the button multiple times quickly, the Serial Monitor will display:Button pressed!
Button pressed!
Button pressed!
- Repeated Behavior:
The program processes each button press event individually and resets thebuttonPressed
flag, ensuring no duplicate outputs for the same press.
Tip: To observe the output, make sure to open the Serial Monitor in the Arduino IDE (click the magnifying glass icon in the top right corner) and set the baud rate to 115200 to match the Serial.begin(115200);
setting in the program.
Conclusion
Using Arduino ESP32 Callback functions for button event handling simplifies the development process for event-driven applications. Callback functions enhance code readability and maintainability while improving event processing efficiency. Although debugging asynchronous functions like ESP32 Callback functions may be challenging, they are invaluable for handling hardware events efficiently.
With this simple example, you can now implement callback functions in your ESP32 projects and streamline button event handling!