ESP32 Pragma Directives Tips | Boost Performance with Optimization Secrets
In embedded development, the ESP32 pragma directives (#pragma) are very useful compiler instructions that help developers pass specific configurations or commands to the compiler. The ESP32 development framework, ESP-IDF (Espressif IoT Development Framework), is based on the GCC compiler, so it supports many ESP32 pragma directives (#pragma). This article will introduce how to use ESP32 pragma directives (#pragma) in ESP32 development, along with examples of their common uses.
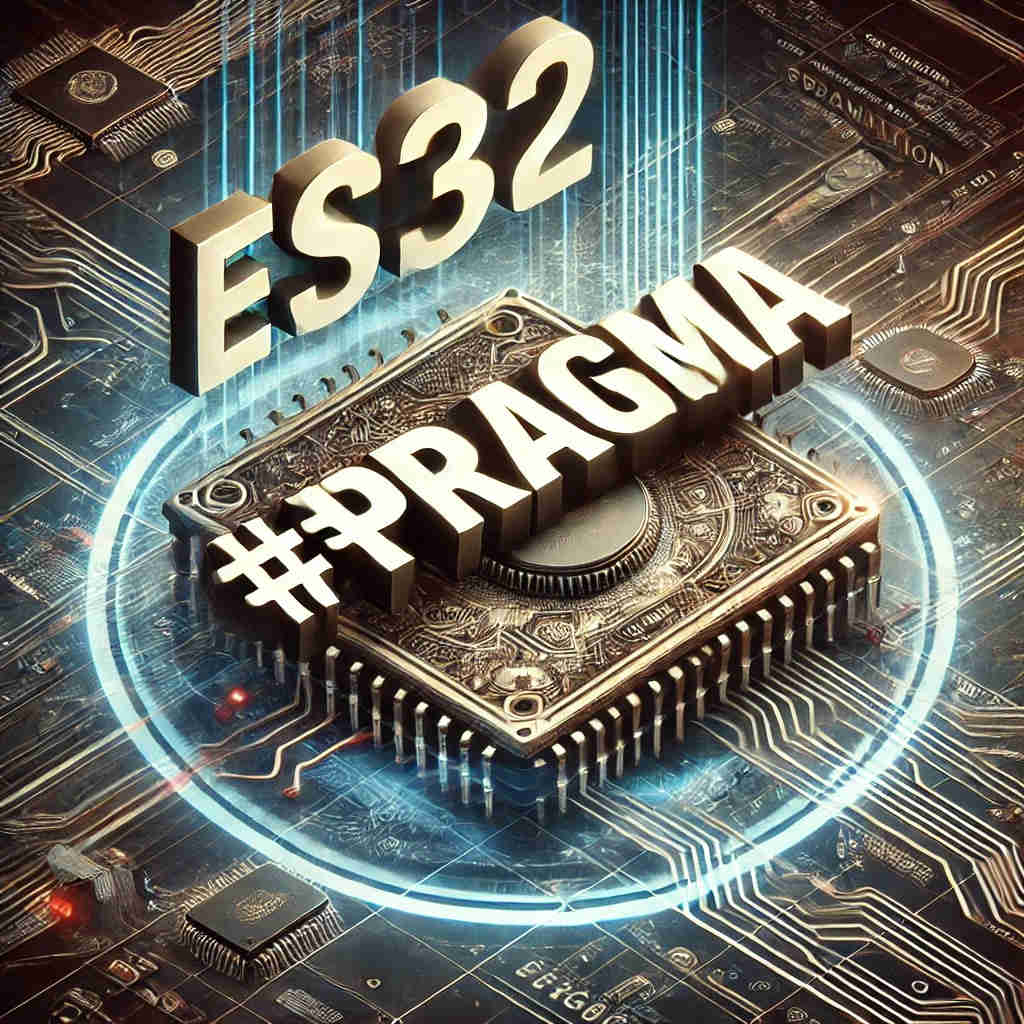
Contents
What are ESP32 Pragma Directives?
#pragma is a preprocessor directive in C/C++ standards that allows passing specific instructions or configurations to the compiler. Its behavior depends on the compiler and platform, so different compilers may support different #pragma directives.
In ESP32 pragma development, #pragma can be used in the following scenarios:
- Optimize code
- Control memory layout
- Enable or disable specific warnings
- Configure compiler behavior
Development Environment
Before starting your programming, make sure to complete the following preparations:
- Install ESP-IDF (version 4.4 or higher): ESP-IDF is the official development framework for programming the ESP32, and it supports multiple operating systems such as Windows, macOS, and Linux.
- ESP32 Development Board: An ESP32 board is required.
Commonly Used ESP32 Pragma (#pragma)
Here are commonly used and supported #pragma directives in ESP32 pragma, based on the functionality of GCC:
#pragma once
This is one of the most common header file protection directives. It tells the compiler that this header file should only be included once to avoid redefinition and unnecessary compiler work.
#pragma once
#pragma GCC optimize
Since ESP32 pragma (#pragma)
uses the GCC compiler, #pragma GCC optimize is a very useful directive. It can be used to specify the compiler optimization level for a function or an entire file.
#pragma GCC optimize ("O3")
GCC supports several optimization levels, and you can choose the appropriate optimization flag based on your needs:
- O0: No optimization. Useful for debugging.
- O1: Basic optimization, removes unnecessary code to increase execution efficiency without increasing compilation time.
- O2: Medium optimization, applies more aggressive optimizations like code inlining, loop optimization, etc.
- O3: High-level optimization, maximizes performance but may increase compilation time.
- Os: Optimizes code size, useful for memory-constrained environments.
- Ofast: Aggressive optimization, skipping some standard specifications (like mathematical precision) to increase runtime speed.
#pragma diagnostic
#pragma diagnostic
is a powerful tool for controlling warning messages during compilation. By using push
, pop
, ignored
, warning
, error
, and default
, developers can flexibly manage warnings, thereby improving code quality and simplifying debugging.
Examples of directives:
- push: Saves the current diagnostic settings; subsequent warning changes will only affect this region and can be restored with
pop
. - pop: Restores the diagnostic settings previously saved by
push
. Typically used withpush
to locally adjust settings. - ignored: Disables specific warnings so they will not appear during compilation.
- warning: Sets a specific diagnostic as a warning. When the warning condition is met, the compiler will display a warning message.
- error: Treats a specific diagnostic as an error. When the warning condition is met, the compiler will treat it as an error and stop compilation.
- default: Resets to the compiler’s default warning settings. Typically used after
ignored
or other settings to reset warnings. - no_ignored: Restores ignored warnings and re-enables them as warnings or errors.
#pragma diagnostic push
#pragma diagnostic ignored "-Wunused-variable" // Disable 'unused variable' warning
void func() {
int unused_var = 10; // No warning here
}
#pragma diagnostic pop // Restore previous diagnostic settings
#pragma pack
The #pragma pack
directive controls the memory alignment of structs, ensuring consistent memory layout and optimizing performance.
Directive parameters:
- #pragma pack(push, 1):
push
saves the current alignment setting and sets the alignment to 1-byte, meaning no padding bytes will be automatically inserted. - #pragma pack(pop):
pop
restores the alignment settings previously saved bypush
, preventing further changes to other struct definitions.
#pragma pack(push, 1) // Save current alignment and set 1-byte alignment
typedef struct {
uint8_t id; // 1 byte
uint16_t value; // 2 bytes (no padding)
uint32_t timestamp; // 4 bytes (no padding)
} PackedStruct;
#pragma pack(pop) // Restore previous alignment settings
#pragma message
#pragma message
is a compiler directive used to output custom messages during the compilation process. It does not affect the execution of the program, but it helps developers display important information, such as debugging tips, version information, or conditional compilation reminders. This is particularly useful in large project management, debugging, or confirming conditional compilation logic.
#pragma message("Your custom message here")
"Your custom message here"
: The custom text message to display in the compiler output window.
Note: Supported by GCC, Clang, and MSVC, but output format may vary slightly across compilers.
Integrated Example
Using various
features in ESP32 IDF:ESP32 pragma directives (#pragma)
#include <stdio.h>
#include "esp_log.h"
// #pragma once: Ensure the header is included only once (typically used in header files)
#pragma once
// #pragma GCC optimize: Set optimization level to O3 (maximum optimization)
#pragma GCC optimize("O3") // O3 - Highest optimization level for performance
// #pragma diagnostic: Control warnings in the code
#pragma diagnostic push
#pragma diagnostic ignored "-Wunused-variable" // Disable unused variable warning
// #pragma message: Display a custom message during compilation
#pragma message("Compiling with O3 optimization and unused variable warning disabled")
// #pragma pack: Set structure alignment to 1-byte boundary
#pragma pack(push, 1) // Set alignment to 1 byte
// Define a packed structure with no padding bytes
typedef struct {
uint8_t id; // 1 byte
uint16_t value; // 2 bytes
uint32_t timestamp; // 4 bytes
} PackedStruct;
#pragma pack(pop) // Restore previous alignment settings
// Main function
void app_main() {
// Example of an unused variable - warning disabled
int unused_var = 10;
// Initialize and populate structure
PackedStruct ps = { 1, 100, 12345678 };
// Print size of packed structure
ESP_LOGI("Example", "Size of PackedStruct: %zu bytes", sizeof(ps));
// Display unused variable (no warning)
ESP_LOGI("Example", "Unused variable (won't show warning): %d", unused_var);
}
Explanation:
- #pragma once: Ensures the header file is only included once to avoid redefinition or unnecessary compilation.
- #pragma GCC optimize(“O3”): Sets the highest optimization level (O3) for maximum performance but may increase compile time.
- #pragma diagnostic:
- push and pop: Save and restore diagnostic settings.
- ignored “-Wunused-variable”: Disables the “unused variable” warning, so the
unused_var
variable doesn’t generate a warning.
- #pragma message(“message”): Outputs a custom message during the compilation process. This is useful for conditional compilation, debugging, or logging compilation settings.
- #pragma pack(push, 1): Sets the alignment to 1-byte, meaning no padding bytes are inserted between struct members. This is useful for low-level hardware programming where memory layout needs to be controlled precisely.
Output
When you run this code on an ESP32, you will see the following log output:
I (123) Example: Compiling with O3 optimization and unused variable warning disabled
I (124) Example: Size of PackedStruct: 7 bytes
I (125) Example: Unused variable (won't show warning): 10
Conclusion
#pragma is a very useful tool that helps you control various behaviors during the compilation process, especially in large projects. It can improve code quality, reduce unnecessary errors, or help developers solve specific problems when needed.
The ESP32 pragma directives (#pragma) in ESP32 depend on the GCC compiler, and the official ESP-IDF documentation does not explicitly list all supported directives. You can refer to the official GCC documentation to learn more about the available #pragma
directives. Common #pragma
directives (such as for optimization control, memory layout, warning management, etc.) are generally available in ESP32.