2025 ESP32 Development Trends | Master C11 Generic to Write Smarter Code!
C11 Generic is a powerful yet often overlooked feature in the C language. It allows developers to dynamically select the appropriate processing logic based on the type of input data, achieving an effect similar to generic programming.
For embedded system development (such as ESP32), this means we can handle multiple data types with more concise code—whether it’s GPIO level control, BLE data parsing, or WiFi message transmission.
With _Generic
, we no longer need to write repetitive functions for each data type. Instead, we can create a unified generic API, making the code more readable and maintainable. This article will guide you through the mechanism of _Generic
, along with practical examples to demonstrate how to implement an efficient generic API on ESP32.
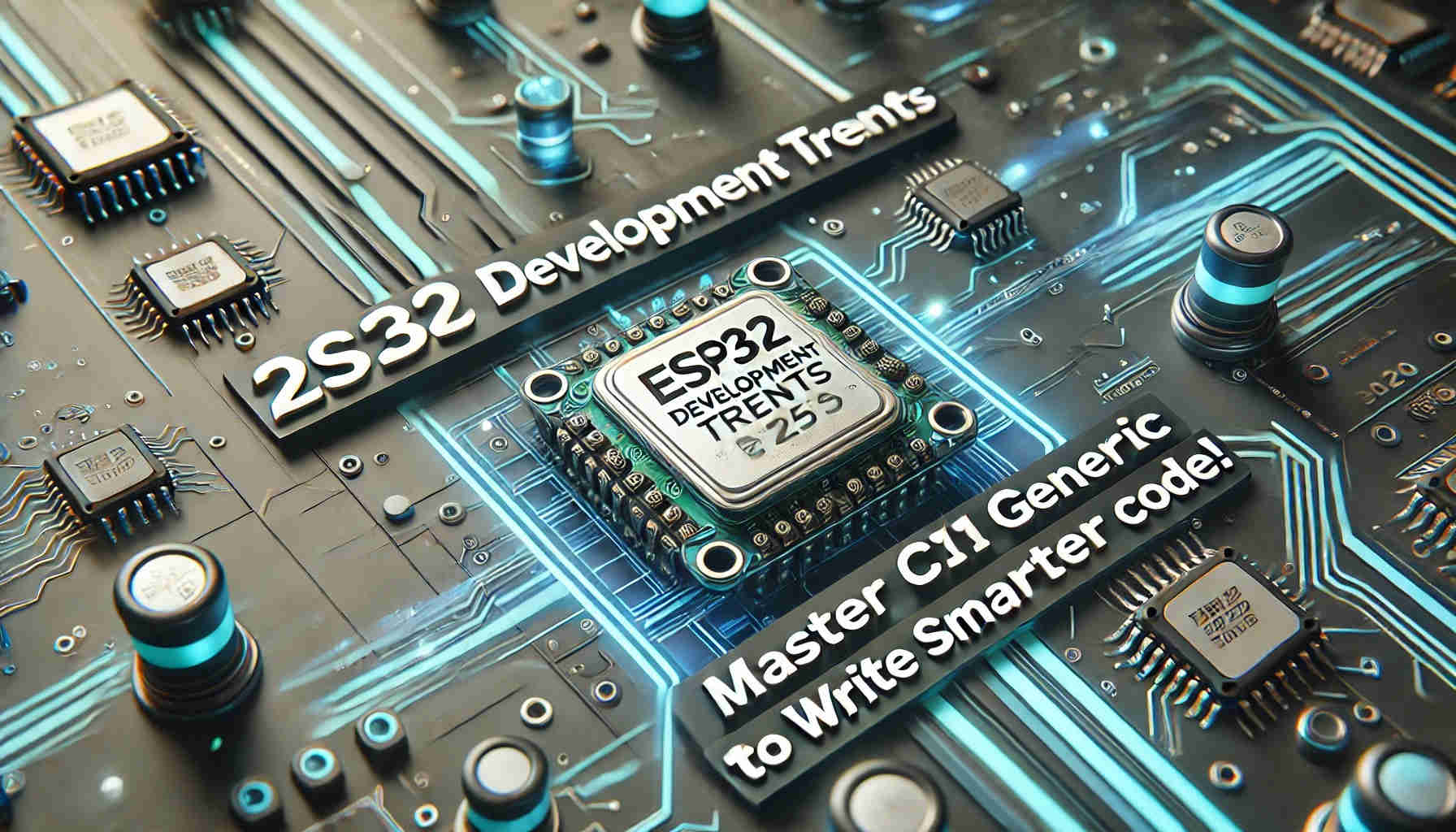
Contents
What is C11 Generic?
_Generic
is a feature introduced in the C11 standard, allowing you to select different code paths based on the type of an expression. Its basic syntax is:
_Generic(expression, type1: result1, type2: result2, ..., default: result_default)
_Generic
determines the type of expression
and selects the corresponding result
. If there is no matching type, it will choose the default
branch.
Advantages of _Generic
- Type Safety: Type checking at compile-time prevents runtime errors.
- Concise Code: Eliminates the need for duplicate code for different data types.
- High Extensibility: New data types can be easily supported by adding corresponding functions in
_Generic
. - Improved Readability: Makes the code logic clearer, reducing the need to worry about specific data types.
Development Environment
Before starting your programming, make sure to complete the following preparations:
- Install ESP-IDF (version 4.4 or higher): ESP-IDF is the official development framework for programming the ESP32, and it supports multiple operating systems such as Windows, macOS, and Linux.
- ESP32 Development Board: An ESP32 board is required.
Using _Generic
for GPIO Control
We can use GPIO operations as an example to illustrate the benefits of _Generic
. In ESP32 development, GPIO operations are very common, such as:
- Setting GPIO mode
- Reading GPIO state
- Controlling GPIO output
These operations often involve different data types (e.g., int
, bool
, or enum
). Using C11 Generic
, we can create a generic API to make GPIO control more concise and flexible.
Example Code: _Generic GPIO Control
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/gpio.h"
#include "esp_log.h"
#define LED_GPIO 2
#define RELAY_GPIO 4
static const char *TAG = "GENERIC_GPIO";
// GPIO Initialization
void gpio_init_output(gpio_num_t pin) {
gpio_config_t io_conf = {
.pin_bit_mask = (1ULL << pin),
.mode = GPIO_MODE_OUTPUT,
.pull_up_en = GPIO_PULLUP_DISABLE,
.pull_down_en = GPIO_PULLDOWN_DISABLE,
.intr_type = GPIO_INTR_DISABLE
};
gpio_config(&io_conf);
}
// Control LED (bool type)
void control_led(bool state) {
gpio_set_level(LED_GPIO, state);
ESP_LOGI(TAG, "LED is now %s", state ? "ON" : "OFF");
}
// Control Relay (int type: 0 or 1)
void control_relay(int state) {
gpio_set_level(RELAY_GPIO, state);
ESP_LOGI(TAG, "Relay is now %s", state ? "Activated" : "Deactivated");
}
// Control PWM (float type: simulate PWM output)
void control_pwm(float duty_cycle) {
int pwm_value = (int)(duty_cycle * 255); // Map 0.0–1.0 to 0–255
gpio_set_level(LED_GPIO, pwm_value > 128 ? 1 : 0); // Simple PWM simulation
ESP_LOGI(TAG, "PWM Duty Cycle: %.2f", duty_cycle);
}
// Generic Macro for GPIO Control
#define gpio_control(x) _Generic((x), \
bool: control_led, \
int: control_relay, \
float: control_pwm \
)(x)
void app_main(void) {
// Initialize GPIOs
gpio_init_output(LED_GPIO);
gpio_init_output(RELAY_GPIO);
// Test Generic GPIO Control
gpio_control(true); // Controls LED: ON
vTaskDelay(pdMS_TO_TICKS(1000));
gpio_control(0); // Controls Relay: OFF
vTaskDelay(pdMS_TO_TICKS(1000));
gpio_control(0.75f); // Controls PWM: 75% Duty Cycle
vTaskDelay(pdMS_TO_TICKS(1000));
gpio_control(false); // Controls LED: OFF
gpio_control(1); // Controls Relay: ON
gpio_control(0.25f); // Controls PWM: 25% Duty Cycle
}
Code Explanation:
GPIO Initialization:
- Uses
gpio_config()
to set up GPIO pins for the LED (GPIO 2) and the relay (GPIO 4).
Control Functions:
control_led()
: Handlesbool
type for turning the LED on or off.control_relay()
: Handlesint
type (0 or 1) for activating or deactivating the relay.control_pwm()
: Handlesfloat
type to simulate PWM control.
Generic Macro gpio_control()
:
- Uses
_Generic
to automatically select the correct control function based on the data type of the argument. - Clean and intuitive API, e.g.,
gpio_control(true)
orgpio_control(0.75f)
.
Output
When you run the program, you should see the following logs in the serial monitor:
I (0) GENERIC_GPIO: LED is now ON
I (1000) GENERIC_GPIO: Relay is now Deactivated
I (2000) GENERIC_GPIO: PWM Duty Cycle: 0.75
I (3000) GENERIC_GPIO: LED is now OFF
I (3000) GENERIC_GPIO: Relay is now Activated
I (3000) GENERIC_GPIO: PWM Duty Cycle: 0.25
Extended Applications of C11 Generic
Besides GPIO control, _Generic
can also be used for:
- I2C/SPI Data Transfer: Selects different transfer methods based on data type.
- Logging System Auto-Formatting: Chooses different formats based on data type.
- Generic Data Processing: Handles sensor data like temperature, humidity, and pressure more efficiently.
Limitations of _Generic
:
- No Runtime Type Checking:
_Generic
works only at compile-time, so it’s not suitable for dynamic type handling. - Requires C11 Support: Ensure ESP-IDF is configured to use the C11 standard (ESP-IDF supports C11 Generic by default).
Conclusion
C11 Generic (_Generic
) is a powerful feature in the C11 standard, especially useful for handling multiple data types efficiently.
With _Generic
, we can create flexible and efficient generic APIs, making code cleaner and easier to maintain. Whether for embedded system development (ESP32) or general applications, C11 Generic can provide significant advantages to your project.