2025 macOS HID – Instantly List Devices with Python, Don’t Waste Time
macOS HID! Have you ever thought about identifying which devices are connected to your computer?
Devices like keyboards, mice, game controllers, and even specialized equipment like barcode scanners or RFID readers are actually all HID (Human Interface Device) devices.
This article will guide you step-by-step on how to list these HID devices on a macOS system using Python and develop in the Visual Studio Code (VSCode) environment.
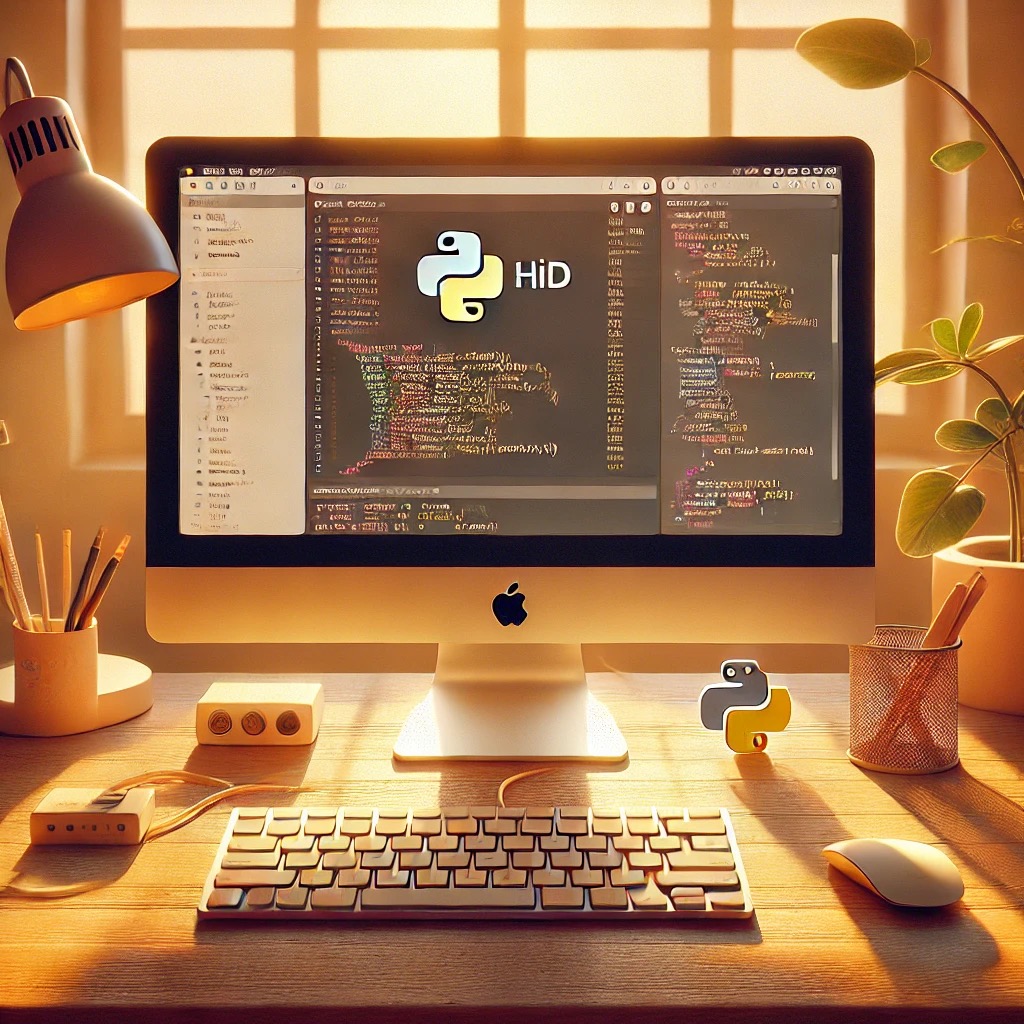
Table of Contents
What is HID?
HID (Human Interface Device) is a type of USB device designed to interact with humans. Common HID devices include:
- Keyboard
- Mouse
- Stylus/Tablet
- Game controllers
- Certain sensors, RFID readers
A key feature of these devices is that they do not require additional drivers—
the system can recognize and support them natively.
Why use Python?
Python is a simple, easy-to-learn language with a powerful ecosystem of libraries.
Using Python to work with HID devices has the following advantages:
- The
hidapi
library supports cross-platform use (Windows, Linux, macOS) - Only a few lines of code are needed to quickly read the device list
- Suitable for rapid prototyping and automated testing
Whether you’re a developer, a maker, or just someone curious about hardware,
Python is an excellent starting point.
Setting Up
- Install Python 3.x or higher.
- Ensure you have access to a terminal or command-line tool to run Python scripts.
Installing Python packages and tools
Before you begin coding, make sure you’ve completed the following setup:
brew install hidapi # Install the C-level hidapi library
pip install hidapi # Install the Python bindings
Creating a project using VSCode
- Create a folder, e.g.,
hid_scanner
- Open the folder in VSCode
- Add a new file:
list_hid_devices.py
macOS HID Code
Here is the full macOS HID source code:
import hid
def list_hid_devices():
devices = hid.enumerate()
if not devices:
print("No HID devices found.")
return
for idx, device in enumerate(devices):
print(f"Device {idx + 1}:")
print(f" Vendor ID: {hex(device['vendor_id'])}")
print(f" Product ID: {hex(device['product_id'])}")
print(f" Manufacturer: {device.get('manufacturer_string')}")
print(f" Product: {device.get('product_string')}")
print(f" Serial Number: {device.get('serial_number')}")
print(f" Path: {device['path'].decode('utf-8') if isinstance(device['path'], bytes) else device['path']}")
print(f" Interface Number: {device.get('interface_number')}")
print("-" * 40)
if __name__ == "__main__":
list_hid_devices()
Code Explanation
The key points of this code are:
- Using
hid.enumerate()
to retrieve a list of all connected HID devices - Using a
for
loop to iterate through and display each device’s information vendor_id
andproduct_id
are fundamental parameters used to identify the devicemanufacturer_string
andproduct_string
typically contain the brand and product namepath
is the internal identifier of the device, relevant for reading and writing operations
This information helps you quickly understand which HID devices are currently connected to your system and can serve as a basis for further communication or control.
macOS HID Devices
Below is a sample output from running list_hid_devices.py
on macOS:
Device 1:
Vendor ID : 0x05ac
Product ID : 0x0250
Manufacturer : Apple Inc.
Product : Apple Internal Keyboard / Trackpad
Serial Number : XYZ1234567
Path : IOService:/AppleACPIPlatformExpert/...
Interface Number: 0
----------------------------------------
Device 2:
Vendor ID : 0x046d
Product ID : 0xc534
Manufacturer : Logitech
Product : USB Receiver
Serial Number : None
Path : IOService:/AppleUSB20HubPort@1a000000/...
Interface Number: 1
----------------------------------------
Device 3:
Vendor ID : 0x1a2b
Product ID : 0x1f2e
Manufacturer : Generic Manufacturer
Product : RFID Reader
Serial Number : A1B2C3D4E5
Path : IOService:/AppleUSB20HubPort@1d000000/...
Interface Number: 2
----------------------------------------
Conclusion
With Python and hidapi
, you can easily list and inspect all macOS HID devices.
This is very helpful for hardware application development, debugging device connections,
or even for automated testing.
Especially on macOS, you don’t need to install any drivers—
it just works out of the box.
As a next step, you can try communicating with HID devices (reading/writing data)
or even build a simple control panel with a GUI.