Master Python Input in 2025 | The Ultimate Guide
Python input handling is one of the most fundamental and indispensable aspects of programming. As one of the most popular programming languages today, Python’s flexibility and powerful features make it a top choice for developers. Whether you’re a beginner just getting started or an experienced programmer, mastering Python input operations is a must-have skill. This guide will take you from the basics to advanced techniques, providing a comprehensive breakdown of Python input handling, along with practical tips to ensure you can effectively use Python in 2025!
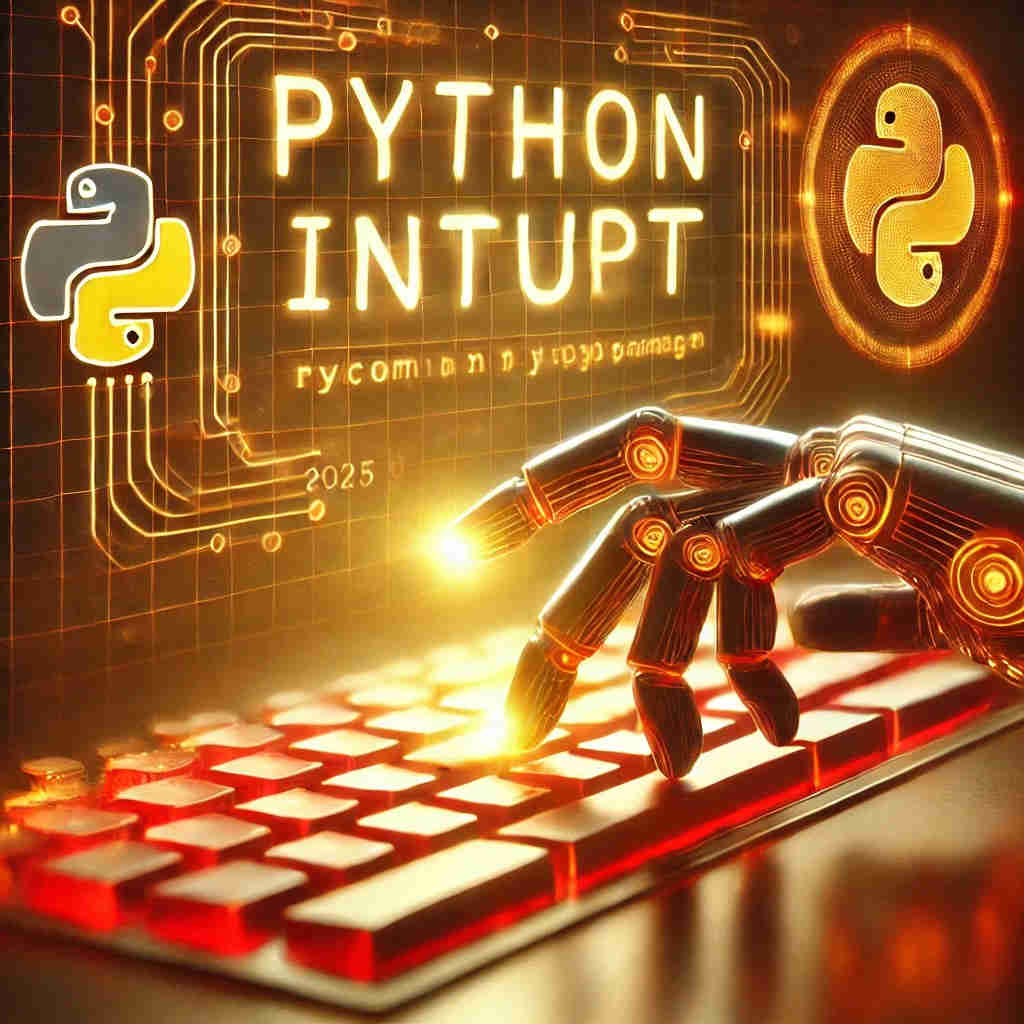
Contents
Python Input Function
Basic Usage :
The most fundamental way to get user input in Python is by using the built-in input()
function. It reads user input from standard input (typically the keyboard) and returns it as a string.
name = input("Enter your name: ")
print(f"Hello, {name}!")
Note: The input()
function always returns data as a string (str
). If another data type is needed, you must explicitly convert it.
Type Conversion
Since input()
always returns a string, you often need to convert the input into other data types such as integers or floats.
age = int(input("Enter your age: "))
height = float(input("Enter your height (in meters): "))
print(f"Age: {age}, Height: {height} meters")
Common Type Conversion Functions
int()
: Converts input to an integerfloat()
: Converts input to a floating-point numberbool()
: Converts input to a boolean value
Space- or Comma-Separated Multiple Values
Handling Space-Separated Input:
If users enter a series of numbers separated by spaces, you can use .split()
to parse them:
numbers = input("Enter numbers separated by spaces: ").split()
numbers = [int(num) for num in numbers] # Convert to a list of integers
print(f"Sum of numbers: {sum(numbers)}")
Handling Comma-Separated Input:
If users enter values like 1,2,3,4,5
, use .split(",")
to process them:
numbers = input("Enter numbers separated by commas: ").split(",")
numbers = [int(num) for num in numbers]
print(f"Maximum number: {max(numbers)}")
Reading Multiple Lines
Sometimes, you need to read multiple lines of input. This can be done using a loop:
print("Enter multiple lines (type 'END' to finish):")
lines = []
while True:
line = input()
if line == "END":
break
lines.append(line)
print("\nYour input:")
print("\n".join(lines))
Reading from a File
When input is coming from a file, you can use the open()
function to read the contents:
with open("data.txt", "r") as file:
content = file.read()
print("File contents:")
print(content)
Reading from Standard Input
sys.stdin.read()
allows you to read all standard input at once. This is useful when handling large amounts of data, such as in competitive programming or batch processing:
import sys
data = sys.stdin.read()
print("Input received:")
print(data)
Reading Command-Line Arguments
For handling script arguments, Python provides the argparse
module:
import argparse
parser = argparse.ArgumentParser(description="Process command-line input")
parser.add_argument("name", type=str, help="Enter your name")
args = parser.parse_args()
print(f"Hello, {args.name}!")
Run the script like this:
python script.py John
Handling Invalid Input
User input may not always be valid. For instance, if a user enters non-numeric characters when an integer is expected, your program might crash. To prevent this, use try-except
to handle exceptions gracefully:
try:
age = int(input("Enter your age: "))
print(f"Your age is: {age}")
except ValueError:
print("Invalid input! Please enter an integer.")
Conclusion
While Python input handling may seem simple at first, there are many useful techniques and best practices that can enhance your programming skills. From the basic input()
function to more advanced methods like argparse
and sys.stdin
, mastering these techniques will make you more efficient and adaptable as a developer.