The Ultimate Guide to Python MQTT in 2025 | Unlocking Powerful IoT Communication
In the rapidly evolving world of the Internet of Things (IoT), Python MQTT (Message Queuing Telemetry Transport) has become one of the most lightweight and efficient messaging protocols. Whether you are building smart home devices, industrial automation systems, or real-time data pipelines, Python MQTT is the preferred solution for seamless communication between devices. We will guide you from scratch to deeply learn how to use Python MQTT with one of the most popular IoT communication protocols to build powerful applications.
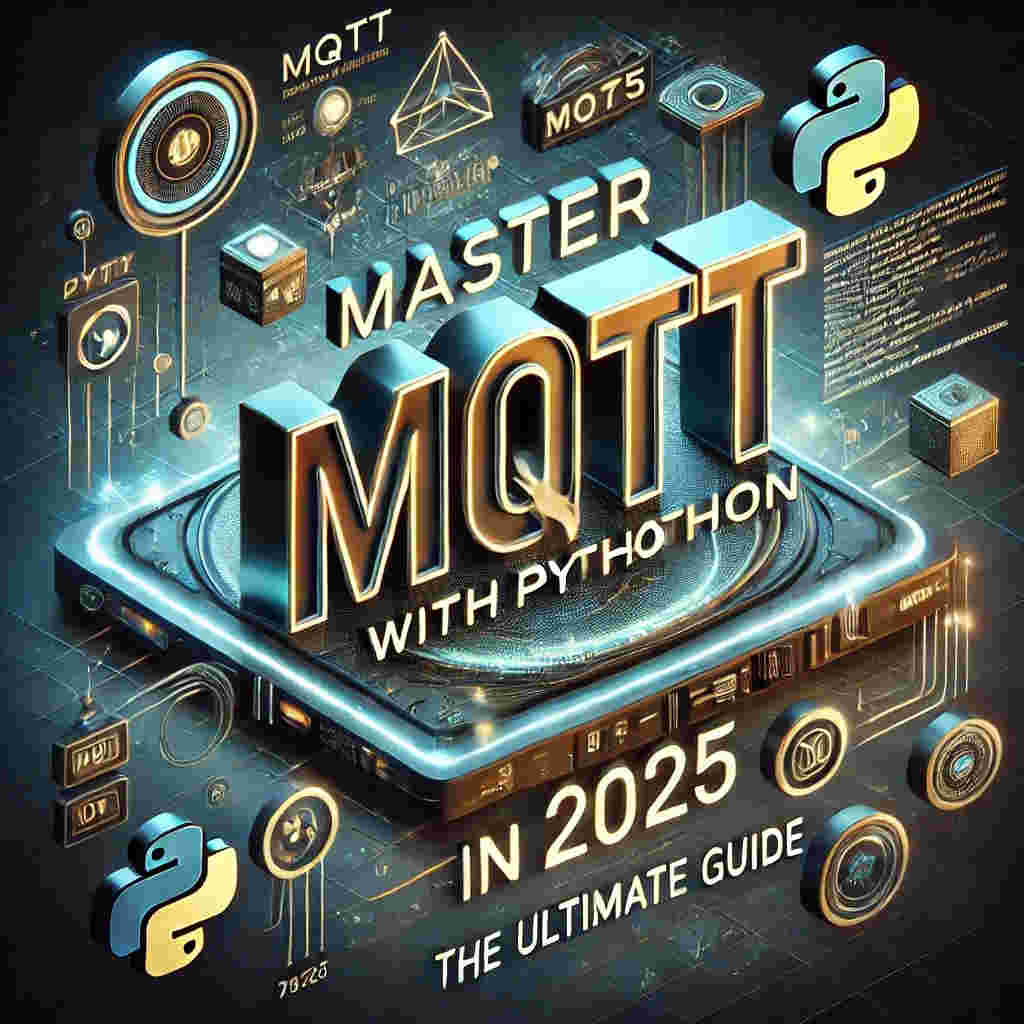
What is MQTT?
This communication protocol is designed to provide fast and lightweight message transmission, especially suitable for environments requiring efficient data exchange. Unlike the traditional request-response model, it adopts a publish-subscribe architecture, allowing devices to send and receive messages asynchronously, making it ideal for real-time data transmission needs. Python MQTT makes implementing this architecture straightforward and efficient.
Key Features:
- Lightweight: Minimal data packet overhead, suitable for low-power devices.
- Broker (Server): Manages message distribution (e.g., Mosquitto).
- Topic: A logical channel for organizing and transmitting messages, similar to a data categorization label.
- Publish-Subscribe Model: Decouples the publisher and subscriber.
- Quality of Service (QoS): Ensures message delivery at different reliability levels.
- Retained Messages: Stores the last message on a topic for new subscribers.
- Last Will and Testament (LWT): Notifies subscribers when a client unexpectedly disconnects.
Python MQTT Development Setup
Before writing the code, we need to install the necessary tools. We will use Python MQTT with a dedicated library to handle all communication tasks, which not only simplifies the development process but also improves efficiency.
Step 1: Install the Required Python Package
To use this communication protocol in Python, we will use the paho-mqtt library. It provides an easy-to-use API to connect to the broker, send messages, and subscribe to topics.
Run the following command in the terminal to install it:
pip install paho-mqtt
If you are using Python 3, run:ython 3,可以執行:
pip3 install paho-mqtt
Step 2: Verify the Installation
After installation, use the following command to confirm that the package is correctly installed:
pip list
Expected output:
Package Version
---------- -------
paho-mqtt 1.6.1
pip 22.3.1
setuptools 65.5.0
This indicates that paho-mqtt has been successfully installed and is ready for use.
Connecting to a Broker
We will use Mosquitto’s free public MQTT test server:
- Server (Broker):
test.mosquitto.org
- Port:
1884
- Username:
rw
- Password:
readwrite
Creating a Subscriber
Next, we will write a simple subscriber program to receive messages from a specific topic.
Subscriber Code Example
import paho.mqtt.client as mqtt
# Callback when connected to the broker
def on_connect(client, userdata, flags, rc):
print("Connected with result code " + str(rc))
client.subscribe("iot_topic/#") # Subscribe to the topic
# Callback when a message is received
def on_message(client, userdata, msg):
print(f"Message from {msg.topic}: {msg.payload.decode('utf-8')}")
# Initialize the client
client = mqtt.Client()
client.username_pw_set("rw", "readwrite")
# Assign callbacks
client.on_connect = on_connect
client.on_message = on_message
# Connect to the broker
client.connect("test.mosquitto.org", 1884, 60)
client.loop_forever()
Code Explanation
on_connect()
: Triggered when the client successfully connects to the broker and automatically subscribes to a specified topic.on_message()
: Called when a message is received, displaying its content.loop_forever()
: Keeps the client running and automatically handles reconnections and message reception.
Creating a Publisher
Now, let’s write a simple publisher program to send messages to the topic we just subscribed to.
Publisher Code Example
import paho.mqtt.client as mqtt
import json
# Message to be sent
msg = "Hello, IoT World!"
# Initialize the client
client = mqtt.Client()
client.username_pw_set("rw", "readwrite")
# Connect to the server
client.connect("test.mosquitto.org", 1884, 60)
# Publish the message
client.publish("iot_topic", json.dumps(msg))
Output (Subscriber Side)
After starting the subscriber program, execute the above publisher program. You should see the following output on the subscriber side:
Connected with result code 0
Message from iot_topic: "Hello, IoT World!"
Advanced Features
Quality of Service (QoS)
MQTT supports three QoS levels:
- QoS 0: At most once (no confirmation required).
- QoS 1: At least once (ensures delivery but may be duplicated).
- QoS 2: Exactly once (ensures delivery without duplication).
Example:
client.publish(topic, message, qos=1)
Retained Messages
Retained messages ensure that new subscribers receive the last message on the topic.
Example:
client.publish(topic, message, retain=True)
Last Will and Testament (LWT)
LWT allows you to specify a message to be sent when the client unexpectedly disconnects.
Example:
client.will_set(topic, "Client disconnected unexpectedly", qos=1, retain=True)
Conclusion
Now you have learned how to use Python MQTT to build a real-time communication system, from basic setup to advanced message publishing and subscription, as well as real-world application scenarios. This lightweight and highly efficient communication architecture is not only suitable for IoT but can also be widely applied to various real-time data processing scenarios using Python MQTT.