Master Python Comments in 2025 | The Ultimate Guide
Comments are an essential part of coding, especially when working on collaborative projects or maintaining long-term codebases. They help developers document the logic, explain functionality, or temporarily disable code. In this guide, we’ll explore Python’s two main ways of adding comments: single-line and multi-line.
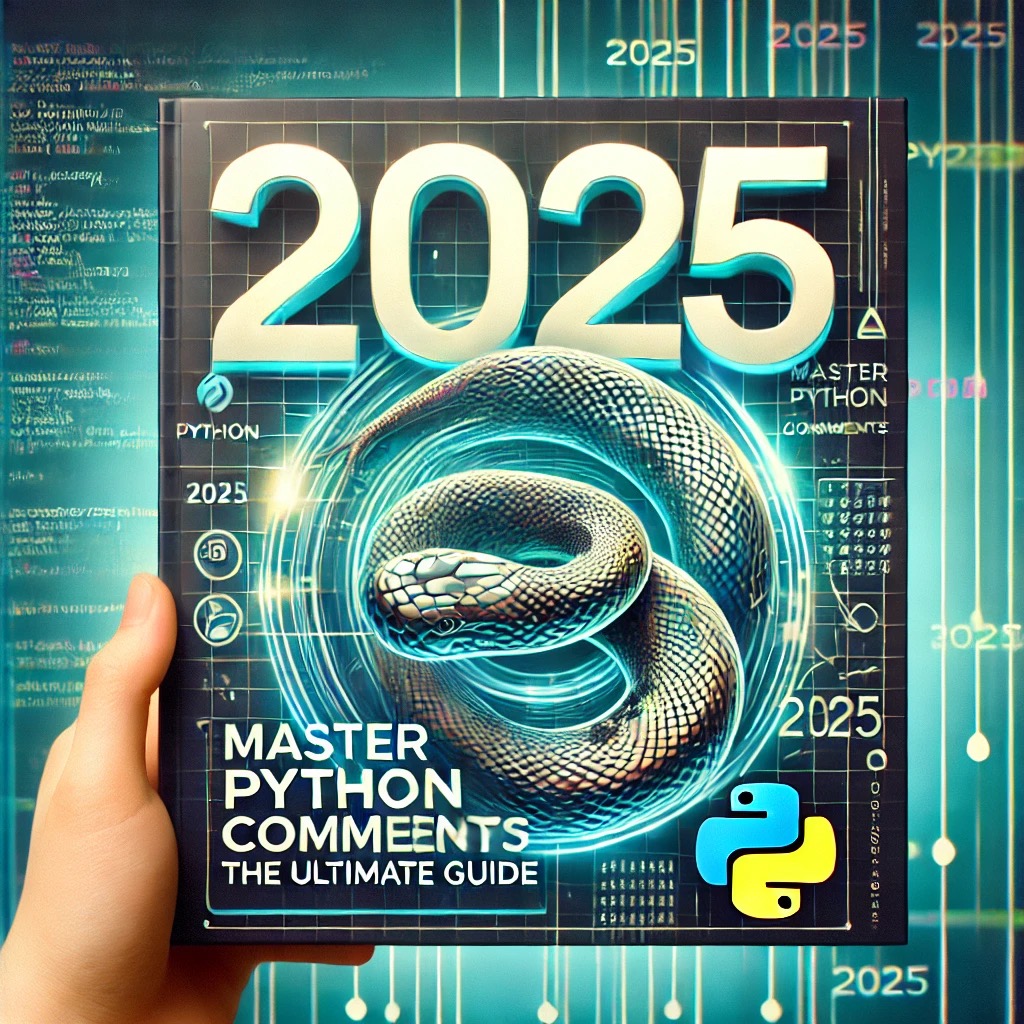
Table of Contents
Single-Line (#
)
Syntax
Single-line in Python are marked with a hash symbol (#
). Any text following the #
on the same line will be ignored by the interpreter.
# This is a single-line comment
Example
Here’s an example of using single-line:
# This line of code will be ignored
# print("Hello World")
print("Hello") # You can also add comments after a line of code
Output
Hello
Common Uses:
- Temporarily disabling a piece of code
- Explaining the logic of specific lines
- Adding notes or special considerations
Multi-Line (""" """
and ''' '''
)
Syntax
Multi-line are created using triple double quotes ("""
) or triple single quotes ('''
). Everything inside these quotes will be ignored by the interpreter.
"""
This is an example of a multi-line comment.
Each line within the quotes is ignored.
"""
or
'''
This is also a multi-line comment.
The usage is identical to triple double quotes.
'''
Example
Here’s an example using multi-line:
"""
This block of code will be ignored:
print("Hello World")
"""
print("Hello SaludPCB") # This line will execute
'''
This block of code will also be ignored:
print("Hello World 1")
print("Hello World 2")
print("Hello World 3")
'''
Output
Hello SaludPCB
Common Uses:
- Documenting the functionality of a large block of code or a module
- Commenting out large sections of test code
- Enhancing code readability with detailed explanations (though for documentation, consider using docstrings)
Best Practices and Tips
- Avoid Over-Commenting: Code should be self-explanatory where possible. Over-commenting can clutter the code and reduce readability.
- Prioritize Clarity: Use concise and clear comments that provide real value. Avoid redundant explanations.
- Follow Standards: Ensure comments follow community guidelines like adding a space after
#
(e.g.,# This is a comment
) as recommended in Python’s PEP 8. - Use Docstrings When Appropriate: For functions, classes, or modules that require extensive explanations, docstrings are preferred over comments.
Conclusion
Effective use of comments is a hallmark of clean and maintainable code. By mastering Python comments, you can write code that is not only functional but also readable and easier to collaborate on. Whether you’re explaining a complex logic, disabling temporary code, or documenting large sections of functionality, knowing when and how to use single-line and multi-line comments is crucial.
In 2025 and beyond, as coding practices evolve, sticking to clear and concise commenting standards will remain essential for every Python developer. Use these tips and techniques to elevate your code quality and make it truly professional.