Master Python Data Types in 2025 | The Ultimate Guide
Understanding data types is fundamental to writing efficient and maintainable code in Python. Whether you’re a beginner learning the basics or an advanced developer optimizing performance, mastering Python’s data types will help you write cleaner, faster, and more reliable code.
In this comprehensive guide, we’ll explore Python data types from the ground up, covering practical examples, advanced techniques, and best practices to help you become a data type expert.
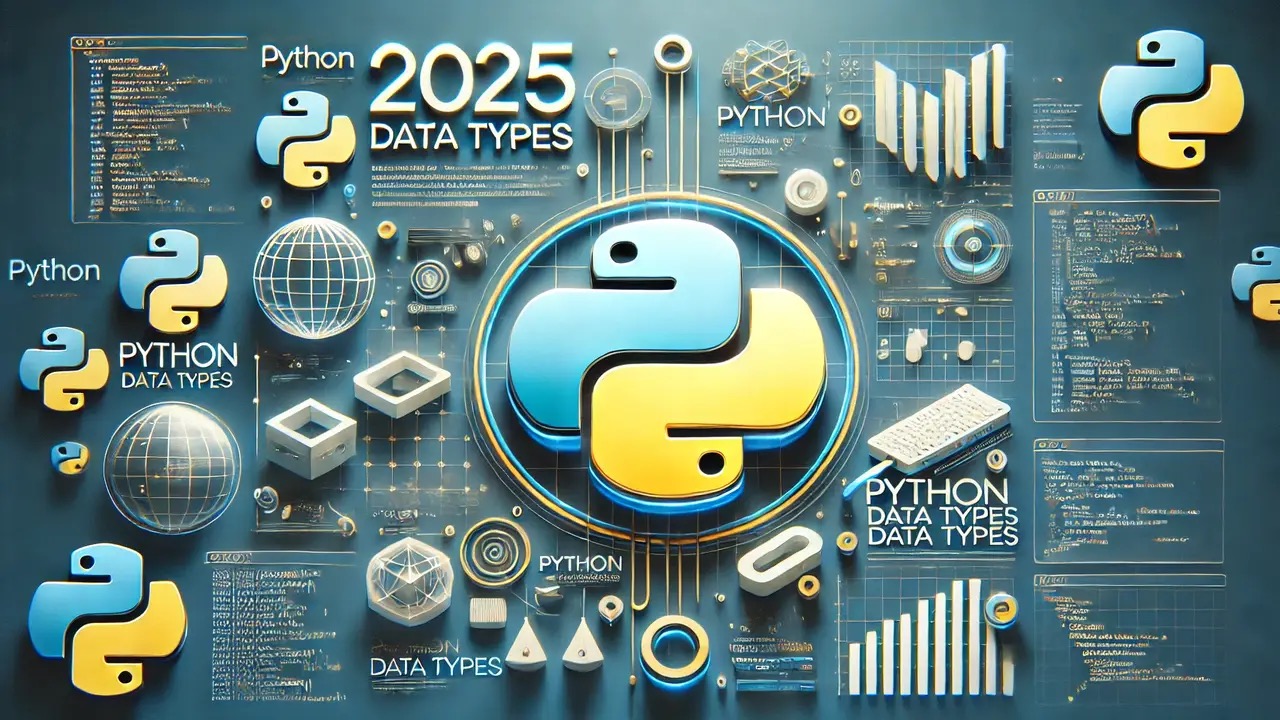
Contents
What Are Python Data Types?
Data types define the kind of data a variable can store and how it can be processed. In Python, you don’t need to explicitly declare data types. Instead, Python uses dynamic typing, which means the data type is automatically determined based on the value assigned to the variable.
Example: Automatic Data Type Inference
# Automatic Data Type Inference
x = 10 # Integer (int)
y = 3.14 # Floating-point number (float)
name = "Alice" # String (str)
is_active = True # Boolean (bool)
# Check data types
print(type(x)) # <class 'int'>
print(type(y)) # <class 'float'>
print(type(name)) # <class 'str'>
print(type(is_active)) # <class 'bool'>
Key Points:
- The
type()
function returns the data type of an object. - Python determines the data type dynamically based on the assigned value.
Data Types in Python
Python offers a variety of built-in data types to handle different kinds of data. These data types can be broadly categorized as follows:
Numeric Types:
int
(Integer): Whole numbers, e.g.,1
,100
,-50
.float
(Floating-point): Decimal numbers, e.g.,3.14
,-0.001
,2.0
.complex
: Numbers with real and imaginary parts, e.g.,2 + 3j
.
a = 42
b = 3.14
c = 2 + 5j
print(type(a), type(b), type(c))
String: A sequence of Unicode characters used to store text data. Strings support various operations like slicing, concatenation, and case conversion.
greeting = "Hello, Python!"
print(greeting.upper()) # Converts to uppercase
print(greeting[0:5]) # String slicing, outputs 'Hello'
Boolean: Represents True
or False
, commonly used in logical operations and conditionals.
is_valid = True
is_empty = False
print(type(is_valid)) # <class 'bool'>
Sequence Types:
list
: A mutable, ordered collection that can hold mixed data types.tuple
: An immutable, ordered collection.range
: Generates a sequence of numbers, commonly used in loops.
# List (mutable)
fruits = ["apple", "banana", "cherry"]
fruits.append("orange")
# Tuple (immutable)
dimensions = (1920, 1080)
# Range
numbers = range(1, 6)
Set Types:
set
: An unordered collection of unique elements, useful for removing duplicates and performing set operations.frozenset
: An immutable version ofset
, ideal when immutability is required.
unique_numbers = {1, 2, 3, 4, 4, 5}
print(unique_numbers) # Duplicates are automatically removed: {1, 2, 3, 4, 5}
Mapping Type:
dict
(Dictionary): A collection of key-value pairs, perfect for storing data with an associated label.
person = {"name": "Alice", "age": 25, "city": "New York"}
print(person["name"]) # Accessing the value associated with the key 'name'
Mutable vs. Immutable Data Types
Python data types are categorized into mutable (can be modified) and immutable (cannot be modified) types.
- Mutable Types:
list
,dict
,set
(can be changed after creation). - Immutable Types:
int
,float
,str
,tuple
(cannot be changed after creation).
Type | Mutability | Element Order | Duplicate Elements | Lookup Efficiency | Use Cases |
---|---|---|---|---|---|
List | Yes | Yes | Allowed | O(n) | Dynamic collections |
Tuple | No | Yes | Allowed | O(n) | Fixed data structures |
Set | Yes | No | Not Allowed | O(1) | Uniqueness checking |
Dict | Yes | Yes* | Unique keys | O(1) | Key-value mappings |
Note: For Dict, the element order has been preserved since Python 3.7, but this behavior is guaranteed from Python 3.8 onwards.
Dynamic Typing and Type Hinting
While Python is dynamically typed, using type hints can improve code readability and maintainability, especially in large projects or collaborative environments.
Example: Type Hinting
def greet(name: str) -> str:
return f"Hello, {name}!"
print(greet("Python"))
Advanced Tip:
Integrate tools like mypy
for static type checking during development to catch type-related errors early.
Type Conversion (Casting) Techniques
Type conversion (also known as type casting) allows you to convert data from one type to another. Python provides built-in functions for type conversion:
# Integer to string
x = 100
str_x = str(x)
# String to integer
num = int("50")
# List to set (remove duplicates)
lst = [1, 2, 3, 3]
unique_set = set(lst)
Note:
If the data is incompatible with the target type, a ValueError
will be raised. Always validate data before conversion.
Advanced Tips: Choosing the Right Data Type
Choosing the appropriate data type enhances both performance and code clarity. Here are some tips:
- For large datasets: Use
set
for fast lookups and de-duplication. - For fixed collections: Use
tuple
to ensure data integrity. - For complex structures: Combine
dict
andlist
to manage nested data efficiently.
Conclusion
Python Data Types are not just basic knowledge; they are the core capability for writing efficient and reliable code. Here are some key best practices:
- Choose the Appropriate Data Type:
Selecting the most suitable data structure based on your needs can enhance both program performance and code readability. - Understand Mutability and Immutability:
Understanding the differences between mutable and immutable types helps prevent unnecessary errors and ensures data consistency in multi-threaded environments. - Leverage Type Hints:
Even in a dynamically typed language, type hints improve code maintainability and enhance error-checking efficiency. - Perform Effective Type Conversion:
Master data conversion techniques to ensure data accuracy and consistency across different scenarios.