Contents
Hardhat Introduction
Hardhat is a Ethereum development environment for compiling, testing, deploying and debugging. Without further ado, let's get started!
Preparations
- Installed the MetaMask and created a account.
- Added the Goerli testnet in MetaMask.
- Obtained the Goerli’s ETH.
- Free 🚰 https://goerlifaucet.com/ ( Need to complete the registration first )
- Installed Node.js
- Obtained the infura’s API_KEY.
- Obtained the MetaMask Wallet’s private key.
Create a Node.js Project
❖ Create a project folder such as "hello_world" and run "npm init -y" as follows (in macOS)...
mkdir hello_world ↩️
cd hello_world ↩️
npm init -y ↩️
When using "npm", you need to install "Node.js" first.
"-y" which means to use the default basic configuration information of the system when the project is initialized.
Install Hardhat Package
❖ Then you need to install Hardhat package in the hello_world project folder as follows...
npm install --save-dev hardhat ↩️
❖ After the installation is complete, you can enter the following command to see the installed files.
ls -a ↩️
. node_modules package.json .. package-lock.json
Create a Hardhat Project
❖ Run "npx hardhat" as follows...
npx hardhat ↩️
888 888 888 888 888 888 888 888 888 888 888 888 888 888 888 8888888888 8888b. 888d888 .d88888 88888b. 8888b. 888888 888 888 "88b 888P" d88" 888 888 "88b "88b 888 888 888 .d888888 888 888 888 888 888 .d888888 888 888 888 888 888 888 Y88b 888 888 888 888 888 Y88b. 888 888 "Y888888 888 "Y88888 888 888 "Y888888 "Y888 👷 Welcome to Hardhat v2.9.9 👷 ? What do you want to do? … ❯ Create a JavaScript project Create a TypeScript project Create an empty hardhat.config.js Quit
choose " Create an empty hardhat.config.js " ↩️
888 888 888 888 888 888 888 888 888 888 888 888 888 888 888 8888888888 8888b. 888d888 .d88888 88888b. 8888b. 888888 888 888 "88b 888P" d88" 888 888 "88b "88b 888 888 888 .d888888 888 888 888 888 888 .d888888 888 888 888 888 888 888 Y88b 888 888 888 888 888 Y88b. 888 888 "Y888888 888 "Y88888 888 888 "Y888888 "Y888 👷 Welcome to Hardhat v2.9.9 👷 ? What do you want to do? … Create a JavaScript project Create a TypeScript project ❯ Create an empty hardhat.config.js Quit
Install OpenZeppelin & Other Packages
❖ Install the openzeppelin and other packages in the hello_world project folder as follows...
npm install --save-dev @openzeppelin/hardhat-upgrades ↩️
npm install --save-dev @nomiclabs/hardhat-ethers ethers ↩️
npm install --save-dev @nomiclabs/hardhat-waffle ↩️
npm install @openzeppelin/contracts ↩️
Install Dotenv Package & Configure .env
❖ Install the Dotenv package as follows...
npm install dotenv ↩️
❖ Create a .env file as follows...
touch .env ↩️
❖ Open .env file and enter a the environment variable as follows...
PRIVATE_KEY=YOUR_PRIVATE_KEY
❗️❗️❗️ "YOUR_PRIVATE_KEY" can be obtained in your MetaMask.
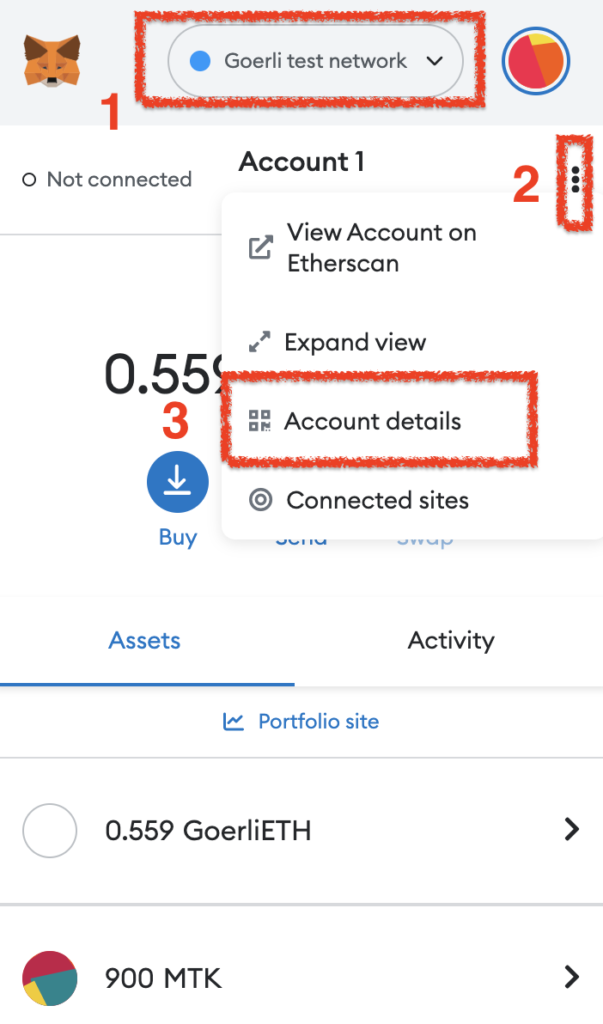
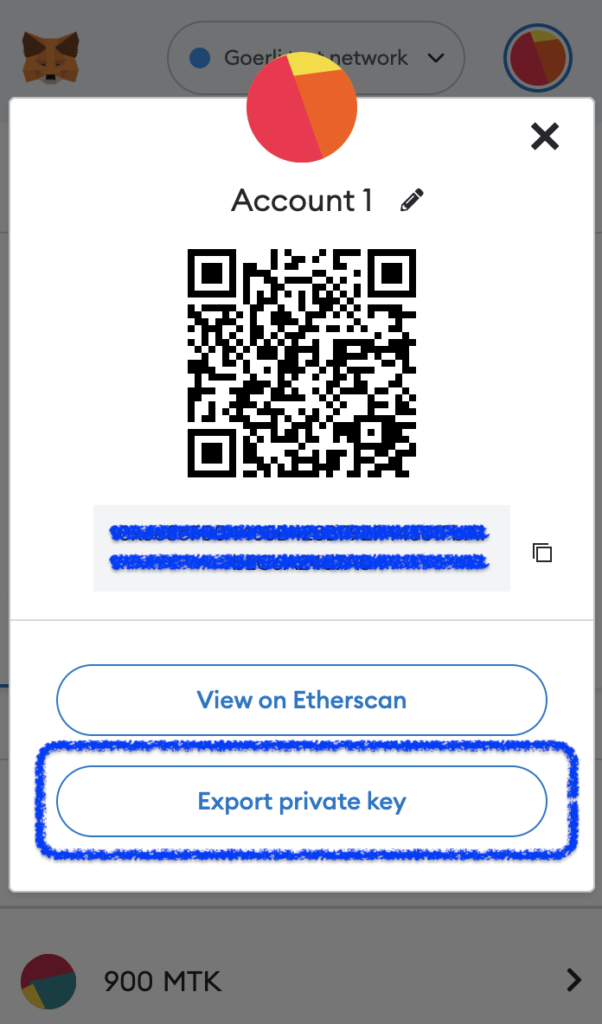
Configure hardhat.config.js
❖ Open your hardhat.config.js file and add code as follows...
require("@nomiclabs/hardhat-waffle");
require('@openzeppelin/hardhat-upgrades');
require('dotenv').config();
/** @type import('hardhat/config').HardhatUserConfig */
module.exports = {
solidity: "0.8.17",
networks:{
goerli:{
url : `https://goerli.infura.io/v3/YOUR-API-KEY`,
accounts: [`0x${process.env.PRIVATE_KEY}`]
}
}
};
❗️❗️❗️ "YOUR-API-KEY", Because we are using "Goerli test network ", so you need create a project in "app.infura.io" before you can get the api_key.
❗️❗️❗️ How to check your "Goerli test network" information as follows...
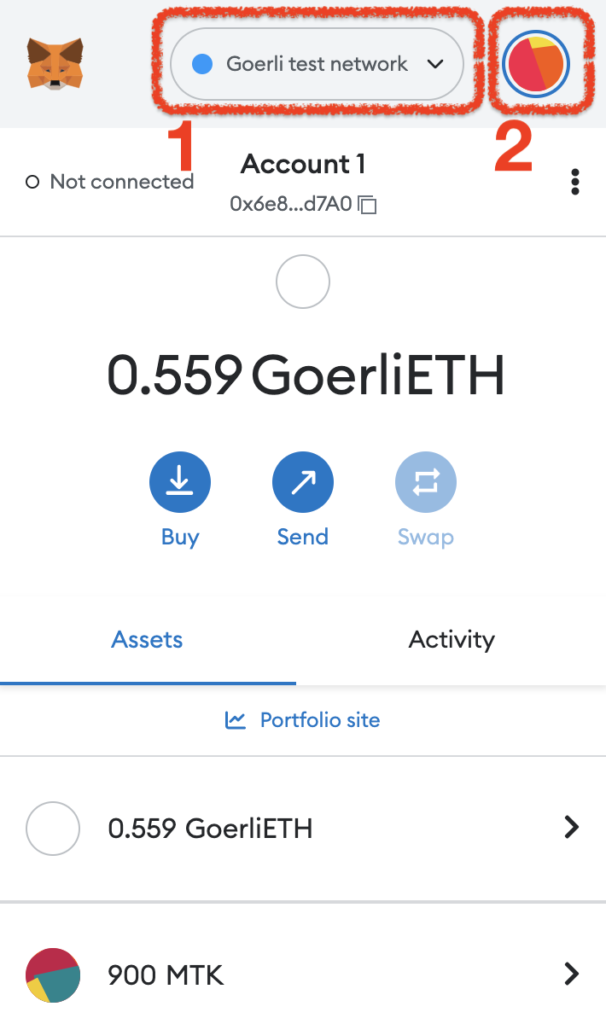
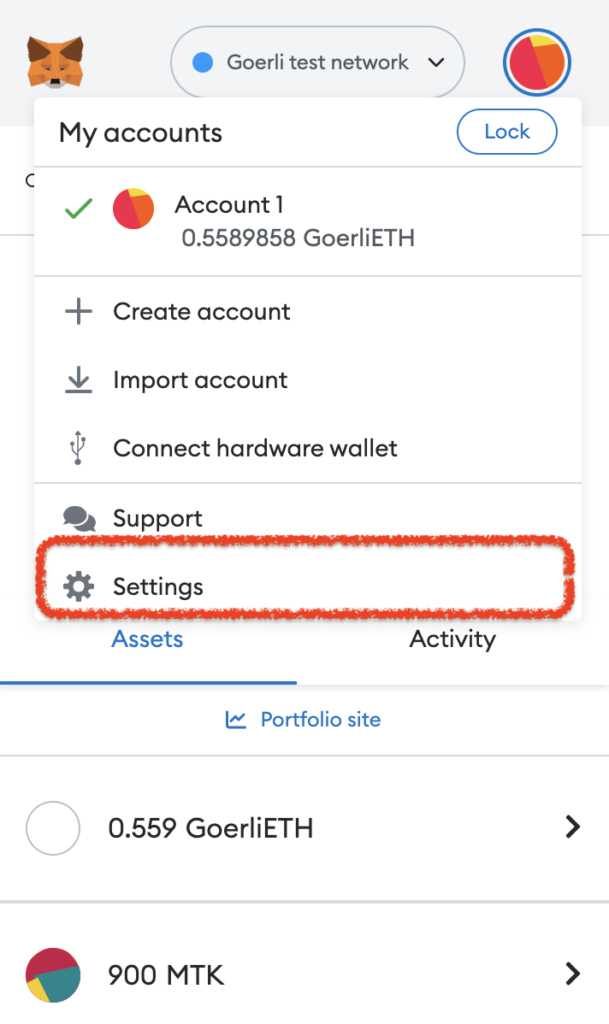
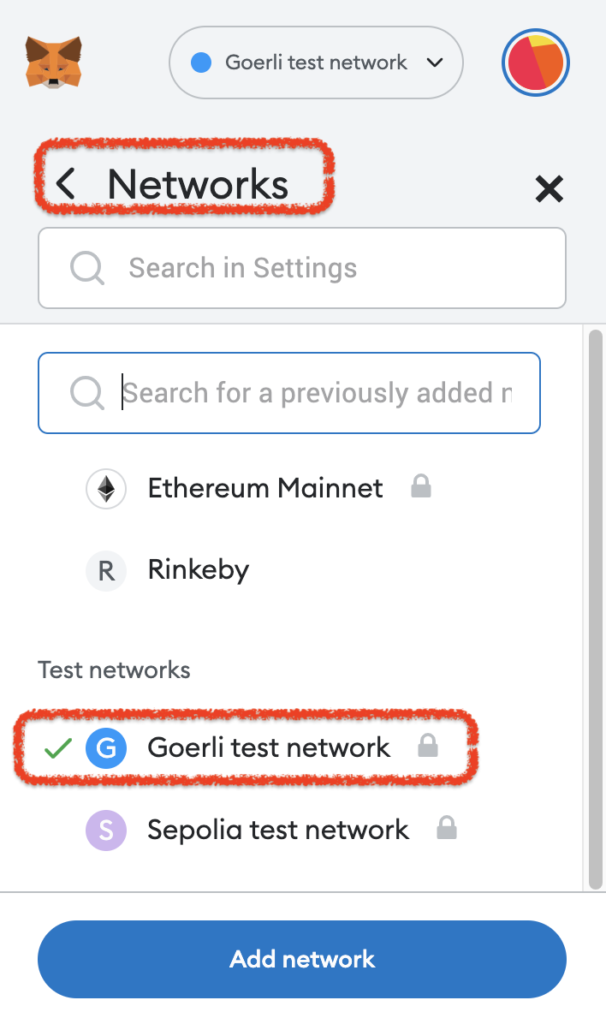
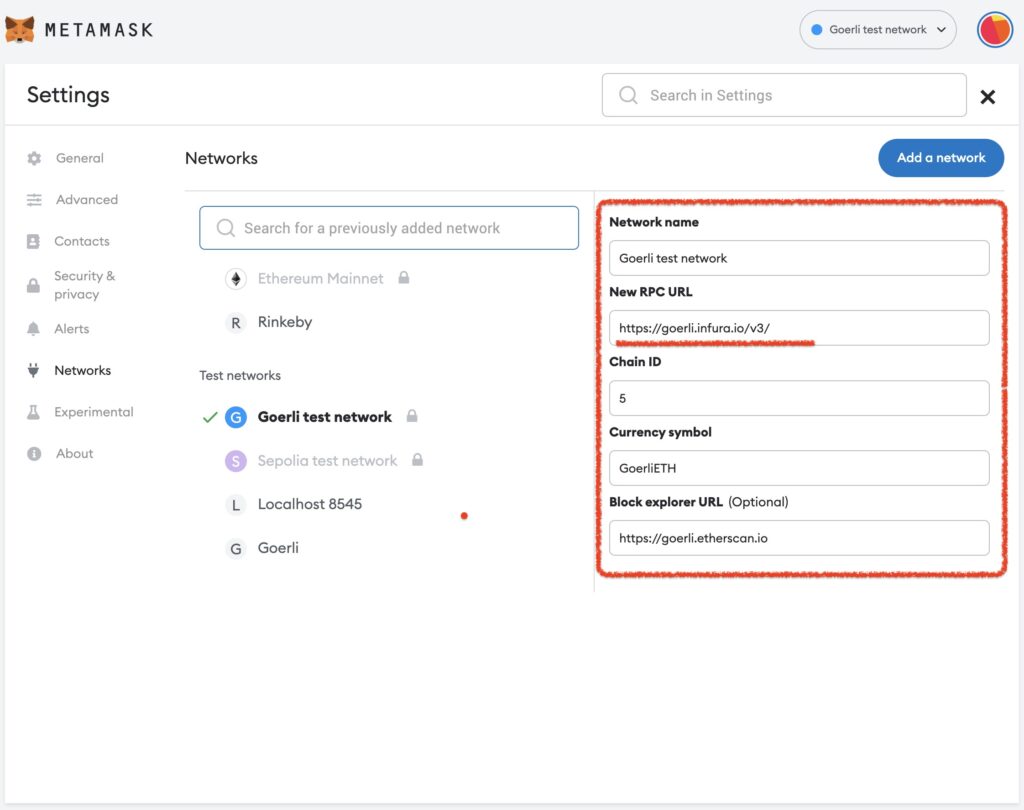
Create a Contracts Folder & ERC-20 Contract
❖ Create a "contracts" folder and "hello_world.sol" file in the hello_world project folder.
mkdir contracts ↩️
cd contracts ↩️
touch hello_world.sol ↩️
❖ Open the "hello_world.sol" file and add code as follows...
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.9;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract hello_world_token is ERC20, Ownable {
constructor() ERC20("hello_world_token", "H_W_TK") {
_mint(msg.sender, 1000 * 10 ** decimals());
}
function mint(address to, uint256 amount)
public onlyOwner {
_mint(to, amount);
}
}
❗️❗️❗️ Contract full name : "hello_world_token". ❗️❗️❗️ Contract symbol name : "H_W_TK".
❖ Compile your contract "hello_world.sol" as follows...
❗️❗️❗️ Back into your project root directory.
cd .. ↩️
npx hardhat compile ↩️
Compiled 6 Solidity files successfully
Deploy Your ERC-20 Contract
❖ Create a "scripts" folder and a "deploy.js" file in the hello_world project folder as follows...
mkdir scripts ↩️
cd scripts ↩️
touch deploy.js ↩️
❖ Open the "deploy.js" file and add code as follows...
async function main() {
const [deployer] = await ethers.getSigners();
console.log("Deploying contracts with the account:",
deployer.address);
const weiAmount = (await deployer.getBalance())
.toString();
console.log("Account balance:",
(await ethers.utils.formatEther(weiAmount)));
// your ERC-20 contract name : hello_world_token
const Token =
await ethers
.getContractFactory("hello_world_token");
const token =
await Token.deploy();
// show your the token address in console window
console.log("Token address:", token.address);
}
// run main
main()
.then(() => process.exit(0))
.catch((error) => {
console.error(error);
process.exit(1);
});
❖ Save and close the "deploy.js" file. ❖ Back into your project root directory. ❖ Run "npx hardhat run scripts/deploy.js --network goerli".
❗️❗️❗️ Need to pay Transaction Fee, so first you need to get Goerli's ETH before you deploy. You can get it for free at https://goerlifaucet.com/.
cd .. ↩️
npx hardhat run scripts/deploy.js --network goerli ↩️
❖ If successful, you will see results as follows...
Deploying contracts with the account: 0x6e8eF004488B428BF92944501FbA1bEC6A24d7A0 Account balance: 0.56278324193332316 Token address: 0xC3E85411B6C734244CB41C40594c85292f63C4a8
Import Your Token to MetaMask
❖ Copy your "Token address :" number e.g."0xC3E85411B6C734244CB41C40594c85292f63C4a8"
❖ Login your MetaMask and choose "Goerli test network".
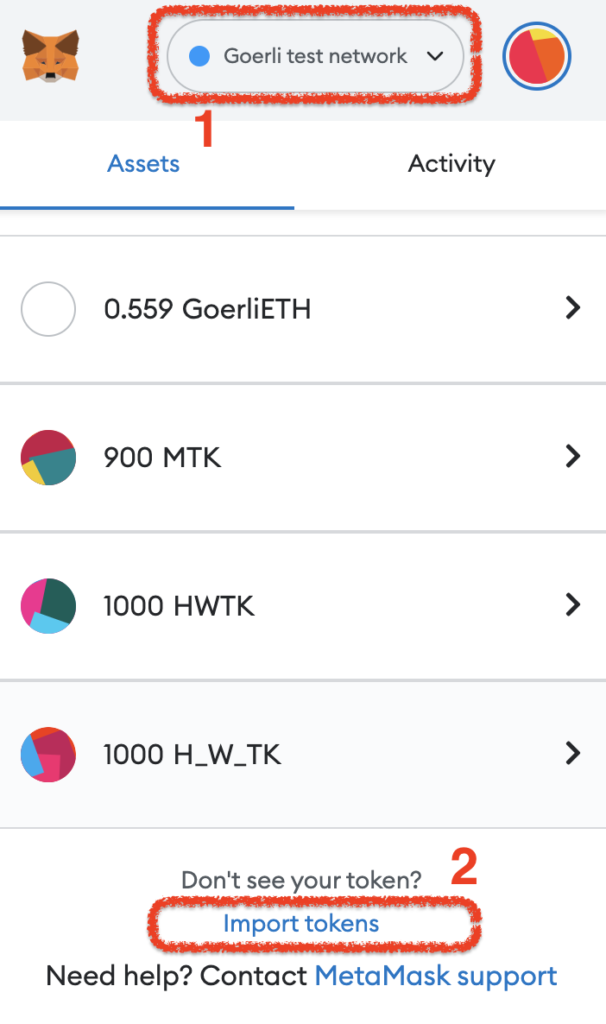
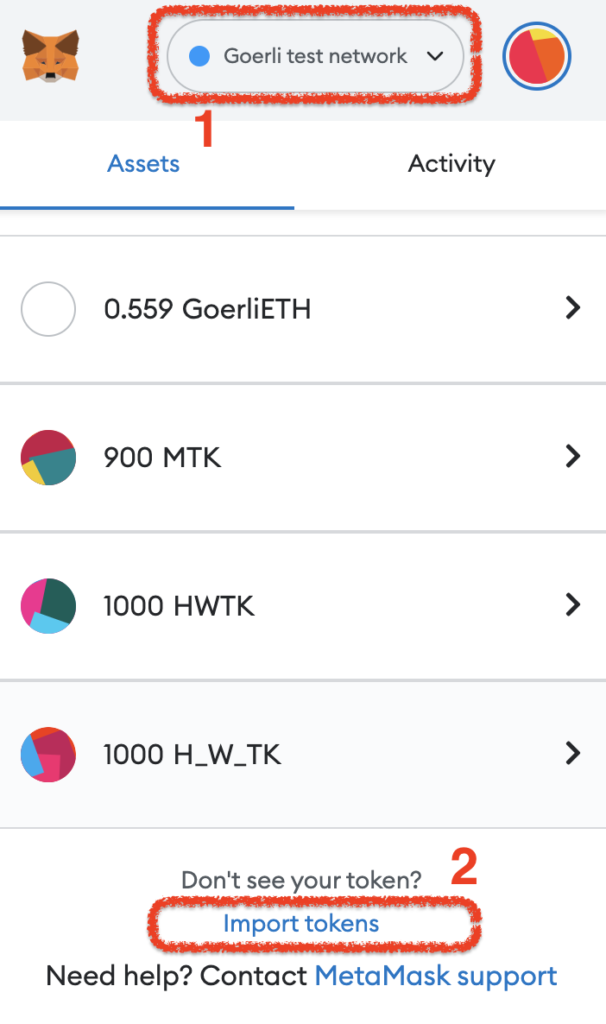
❖ Paste your "Token address" to the "Token contract address" field and click the "Add custom token".
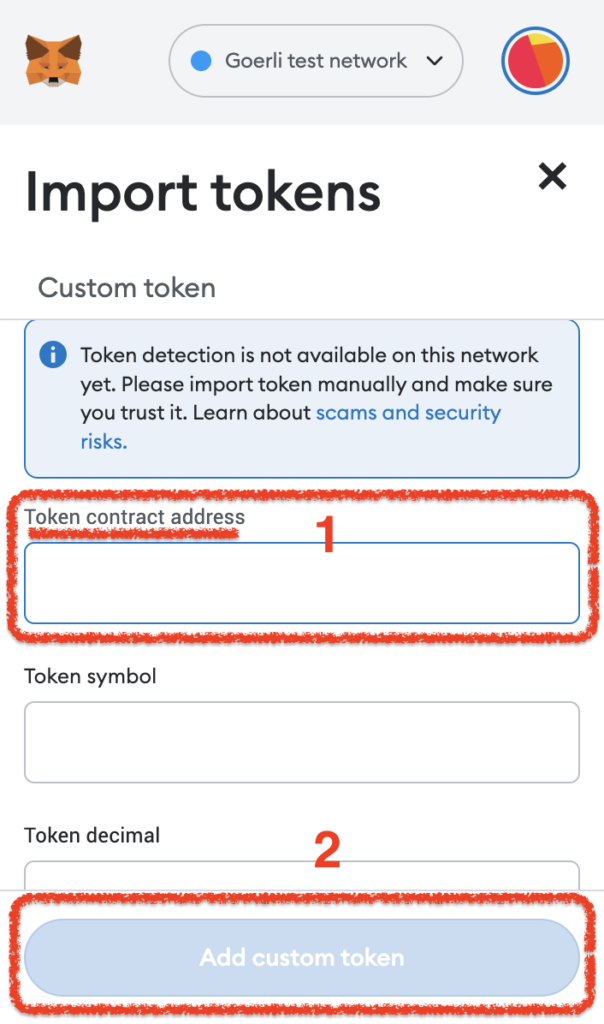
🎉 Congratulations finally finished.
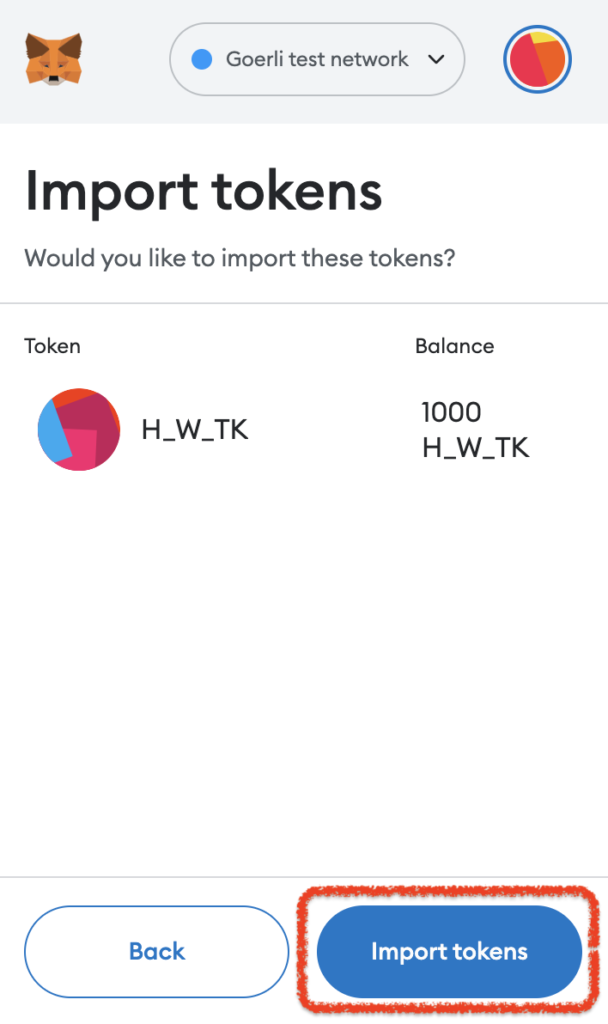
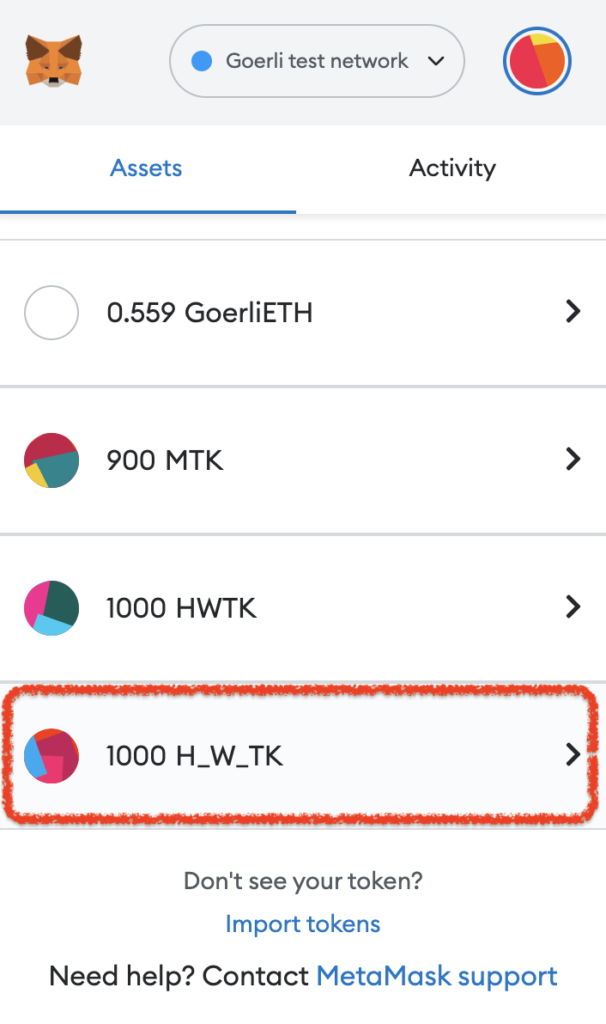