打造 AI 按鈕 | Arduino ESP32 解鎖 Multi-Function Key 多功能按鍵操作
Multi-Function Key(多功能按鍵) 是現代智能設備中的一個重要特性,它不僅能執行基本的開關操作,還能根據不同的點擊方式實現多種功能。使用 Arduino ESP32,你可以輕鬆打造一個 Multi-Function Key,讓你的按鈕更加智能,能夠支持短按、長按、雙擊等多種交互方式。這篇文章將教你如何利用 Arduino ESP32 實現這樣的 Multi-Function Key,並探索它的應用場景和設計原理。
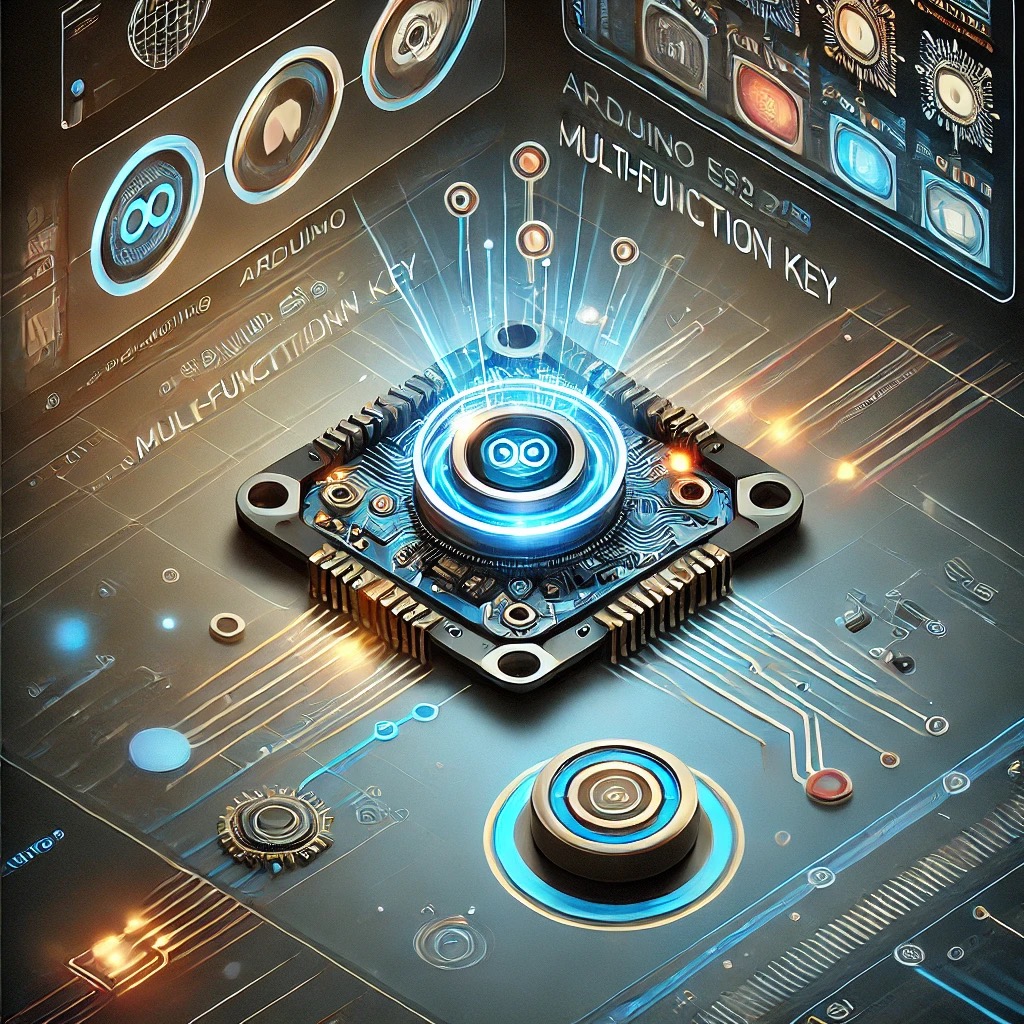
內容
簡介
當我們談論 多功能按鍵(Multi-Function Key) 和 事件(Events) 時,主要指的是通過不同的按鍵操作(如短按、長按、雙擊等)來觸發不同的功能或行為。多功能按鍵 是一種讓按鈕具有更多操作方式的設計,而 事件 是這些操作方式所觸發的具體行為或反應。通过這種設計,用戶可以通過簡單的按鍵操作來控制更多功能,從而提升設備的智能性和交互性。
什麼是 Multi-Function Key?
Multi-Function Key(多功能按鍵)是指一個按鍵,通過不同的點擊方式或長短按等動作,能夠實現不同的功能或操作。例如:
- 雙擊:觸發某一特定操作
- 長按:啟動某種功能或切換模式
- 短按:快速執行基本操作
這種設計讓按鍵擁有多種可能性,而不是只用來開啟或關閉某個設備。
開發環境
在開始編程之前,請確保已完成以下準備工作:
- 安裝 Arduino IDE 和 ESP32 開發環境。
- ESP32 開發板。
實現多功能按鍵的基本原理
- 按鍵行為的識別:短按(Single Click),長按(Long Press),雙擊(Double Click),三擊(Triple Click),連續點擊。
- 按鈕間隔時間:這是判斷連擊操作是否為雙擊、三擊等的關鍵。比如兩次按下之間的時間超過500毫秒,就可以認為是一次單擊,反之則視為雙擊。
- 時間管理與判斷邏輯:為了區分這些行為,時間是關鍵的因素。具體來說,按鈕的按下時間、釋放時間以及按鈕之間的間隔時間都需要進行管理。
- 防抖與去噪 : 按鈕的物理結構可能會導致按鍵輸入在按下或釋放時產生一些“抖動”(即短時間內的多次開關切換)。這些抖動可能會被誤識別為額外的按鈕事件,從而導致錯誤的反應。因此,需要在硬體和軟體中進行防抖處理。
- 硬體中斷與輪詢 : 可用兩種常見的方法來檢測按鈕事件。
- 事件處理 : 一旦按鍵行為被識別,接下來的任務是處理這些事件。這通常涉了 事件分類,如單擊、雙擊、長按等,系統將對事件進行分類並決定對應的操作。觸發相應操作,每種按鈕事件都會對應一個或多個操作。例如,短按可能觸發燈光開關,而長按可能啟動某個模式,雙擊可能用來調整音量。
程式碼實現
以下是一個程式碼的範例,示範如何使用 Arduino 和 ESP32 撰寫程式,讓按鈕實現不同功能:
#define BUTTON_PIN 2 // Define the pin connected to the button
// Enumeration for different button events
enum ButtonEvent { NONE, SINGLE_CLICK, DOUBLE_CLICK, TRIPLE_CLICK, LONG_PRESS };
// Volatile variables to store the current event and button states
volatile ButtonEvent currentEvent = NONE; // Current button event
volatile unsigned long lastPressTime = 0; // Time when the button was last pressed
volatile unsigned long pressDuration = 0; // Duration the button was held
volatile int clickCount = 0; // Number of consecutive clicks
// Timing thresholds
unsigned long debounceTime = 50; // Debounce time to prevent false triggers (50 ms)
unsigned long longPressThreshold = 1000; // Threshold for a long press (1000 ms)
unsigned long clickTimeout = 300; // Time window for detecting double/triple clicks (300 ms)
// Interrupt service routine for handling button press and release
void IRAM_ATTR handleButtonInterrupt() {
static unsigned long lastInterruptTime = 0; // To track the last interrupt time
unsigned long interruptTime = millis(); // Get the current system time
if (interruptTime - lastInterruptTime > debounceTime) { // Debounce check
if (digitalRead(BUTTON_PIN) == LOW) { // Button is pressed (LOW due to INPUT_PULLUP)
lastPressTime = interruptTime; // Record the press time
} else { // Button is released
pressDuration = interruptTime - lastPressTime; // Calculate the duration of the press
if (pressDuration >= longPressThreshold) { // Check if it is a long press
currentEvent = LONG_PRESS; // Set the event to LONG_PRESS
} else {
clickCount++; // Increment the click count for short presses
}
}
}
lastInterruptTime = interruptTime; // Update the last interrupt time
}
void setup() {
pinMode(BUTTON_PIN, INPUT_PULLUP); // Set button pin as input with an internal pull-up resistor
attachInterrupt(digitalPinToInterrupt(BUTTON_PIN), handleButtonInterrupt, CHANGE);
// Attach an interrupt to the button pin to handle both press and release events
Serial.begin(115200); // Initialize serial communication for debugging
}
void loop() {
static unsigned long lastCheckTime = 0;
// Check if click events need to be processed
if (millis() - lastPressTime > clickTimeout && clickCount > 0) {
switch (clickCount) {
case 1: currentEvent = SINGLE_CLICK; break; // Single click
case 2: currentEvent = DOUBLE_CLICK; break; // Double click
case 3: currentEvent = TRIPLE_CLICK; break; // Triple click
}
clickCount = 0; // Reset the click count
}
// Print the current event
if (currentEvent != NONE) {
printEvent(currentEvent); // Print the detected event
currentEvent = NONE; // Reset the event after processing
}
}
// Function to print the detected button event to the Serial Monitor
void printEvent(ButtonEvent event) {
switch (event) {
case SINGLE_CLICK: Serial.println("Single Click"); break;
case DOUBLE_CLICK: Serial.println("Double Click"); break;
case TRIPLE_CLICK: Serial.println("Triple Click"); break;
case LONG_PRESS: Serial.println("Long Press"); break;
case NONE: break; // No event to process
}
}
程式碼解說
定義按鈕引腳和事件類型 ButtonEvent
枚舉包含了不同的事件類型:
NONE
(無事件)SINGLE_CLICK
(單擊)DOUBLE_CLICK
(雙擊)TRIPLE_CLICK
(三擊LONG_PRESS
(長按)
全局變數定義
currentEvent
用來儲存當前的按鈕事件。lastPressTime
和pressDuration
用來追蹤按下按鈕的時間,以便識別長按和短按。clickCount
用來計算按鈕被點擊的次數。debounceTime
用於防止按鈕抖動引起誤觸。longPressThreshold
設置長按的時間閾值(此處是 1000 毫秒,即 1 秒)。clickTimeout
設定雙擊或三擊的時間窗口,若按下之間的時間小於 300 毫秒,則認為是雙擊或三擊。
中斷處理函數 handleButtonInterrupt()
millis()
用於獲取當前的系統時間(以毫秒為單位)。- 防抖處理:如果按鈕按下或釋放的間隔時間小於
debounceTime
,則忽略這次事件。 - 如果按鈕被按下,記錄按下時間 (
lastPressTime
)。 - 如果按鈕被釋放,計算按下的持續時間
pressDuration
,並根據持續時間來識別是否為長按。如果不是長按,則計算按下的次數(點擊次數)。
初始化設置在 setup()
函數中:
- 設定按鈕引腳為輸入,並啟用內部上拉電阻。
attachInterrupt()
用來將按鈕狀態變化時的中斷與handleButtonInterrupt
函數綁定。- 初始化序列通訊,以便在終端打印按鈕事件。
主循環處理 loop()
函數是 Arduino 的主循環:
- 判斷
clickCount
(按鈕被點擊的次數)是否達到雙擊或三擊的時間窗口(clickTimeout
)。若達到時間限制,則根據clickCount
設定事件類型。 - 使用
printEvent()
函數打印按鈕事件。
輸出
以下是一段串列監視器的可能顯示輸出,模擬多種按鍵事件的觸發:
Single Click
Double Click
Triple Click
Long Press
Single Click
結論
使用 Arduino ESP32,你可以輕鬆設計和實現一個多功能按鈕,並讓你的設備擁有更多功能和智能操作。這樣的設計不僅增加了使用樂趣,還讓創意與實現變得更加有價值!希望這篇文章能幫助你成功打造屬於自己的 AI 按鈕。式應用中。透過這種方法,我們能夠實現穩定的按鍵輸入,提升系統的穩定性和可靠性。